Certainly! Python is an interpreted, high-level, general-purpose programming language.
We have learned that Python is an interpreted language.
Well, what does it mean?
It means that we actually run another program that is responsible for reading our source code and running it top to bottom.
In Python, this “another program” we just referred to is called an Interpreter. Simply put, an Interpreter is a kind of program that executes other programs.

What is the Need of Interpreter if we already had Compiler?
The real need of interpreter comes where compiler fails to satisfy the software development needs. The compiler is a very powerful tool for developing programs in a high-level language.
However, there are several demerits associated with the compiler. If the source code is huge in size, then it might take hours to compile the source code. Which will significantly increase the compilation duration. Here interpreter comes handy and can cut this huge compilation duration. Interpreters are designed to translate single instruction at a time and execute them immediately.
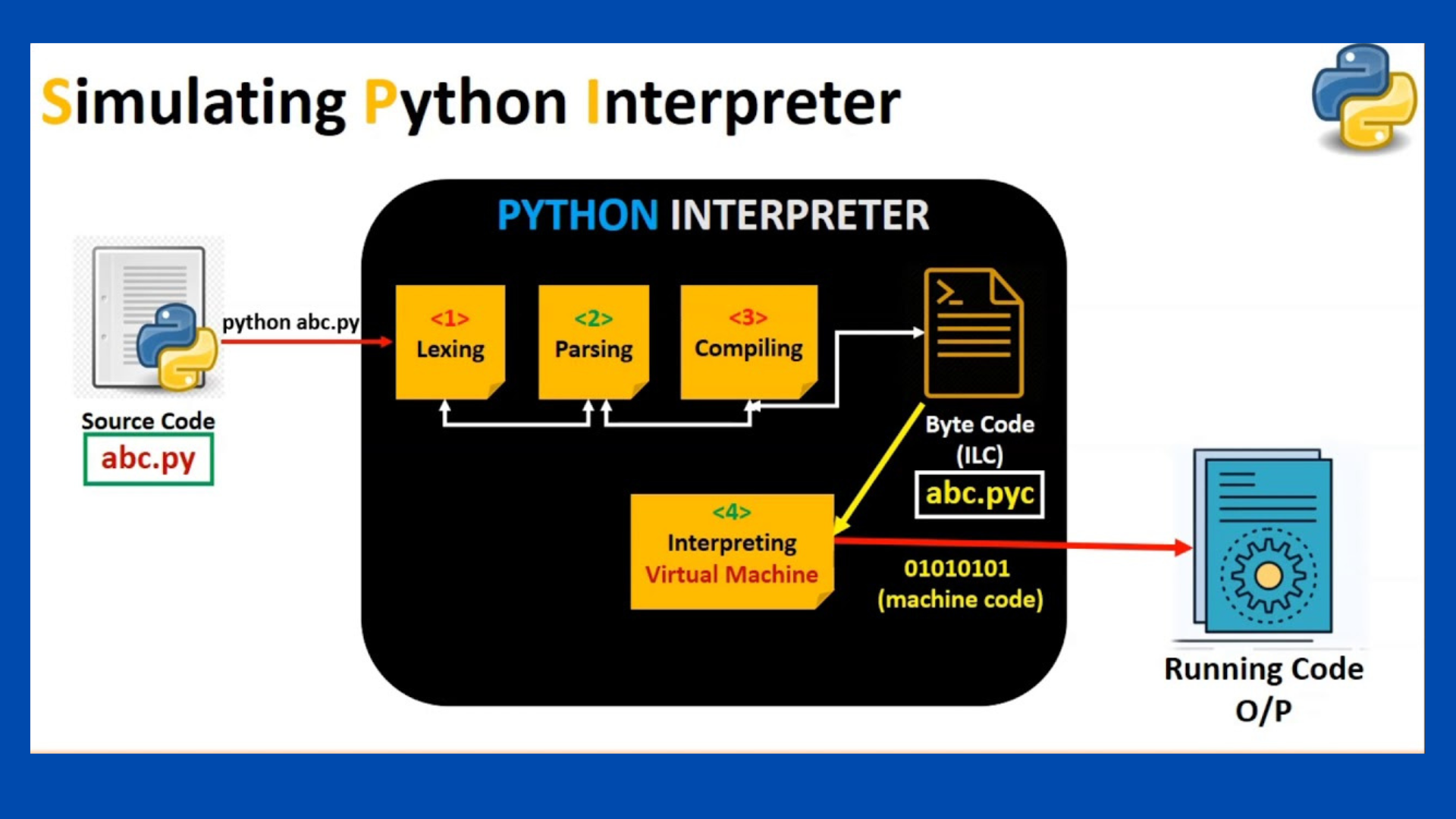
Here's an overview of its workings, step by step:
1.Source Code :
You start by writing your Python code in a text file with a .py
extension.
2.Lexical Analysis (Tokenization):
The Python interpreter first performs lexical analysis, where the source code is broken down into a sequence of tokens. Tokens are the smallest units of meaning, such as keywords, identifiers, literals, and operators.
3.Syntax Parsing (Abstract Syntax Tree - AST):
The tokenized code is then parsed into an Abstract Syntax Tree (AST). The AST represents the hierarchical structure of the code, capturing the relationships between different elements.

4.Intermediate Code (Bytecode) Generation:
The Python compiler generates an intermediate code known as bytecode from the AST. Bytecode is a low-level, platform-independent representation of the code that can be executed by the Python Virtual Machine (PVM).

5.Code Optimization:
It improves time and space requirement of the program. For doing so, it eliminates the redundant code present in the program.
6.Execution by Python Virtual Machine (PVM):
The bytecode is executed by the Python Virtual Machine (PVM). The PVM is an interpreter that translates the bytecode into machine code or executes it directly, depending on the implementation. CPython, the reference implementation, compiles bytecode into machine code on-the-fly using a Just-In-Time (JIT) compiler.
Python Virtual Machine is the runtime engine of Python. It goes through your byte code instructions one by one and carries out the operations stated in those instructions.
Technically, the execution of your byte code by PVM is the ‘Python Interpreter’ last step.
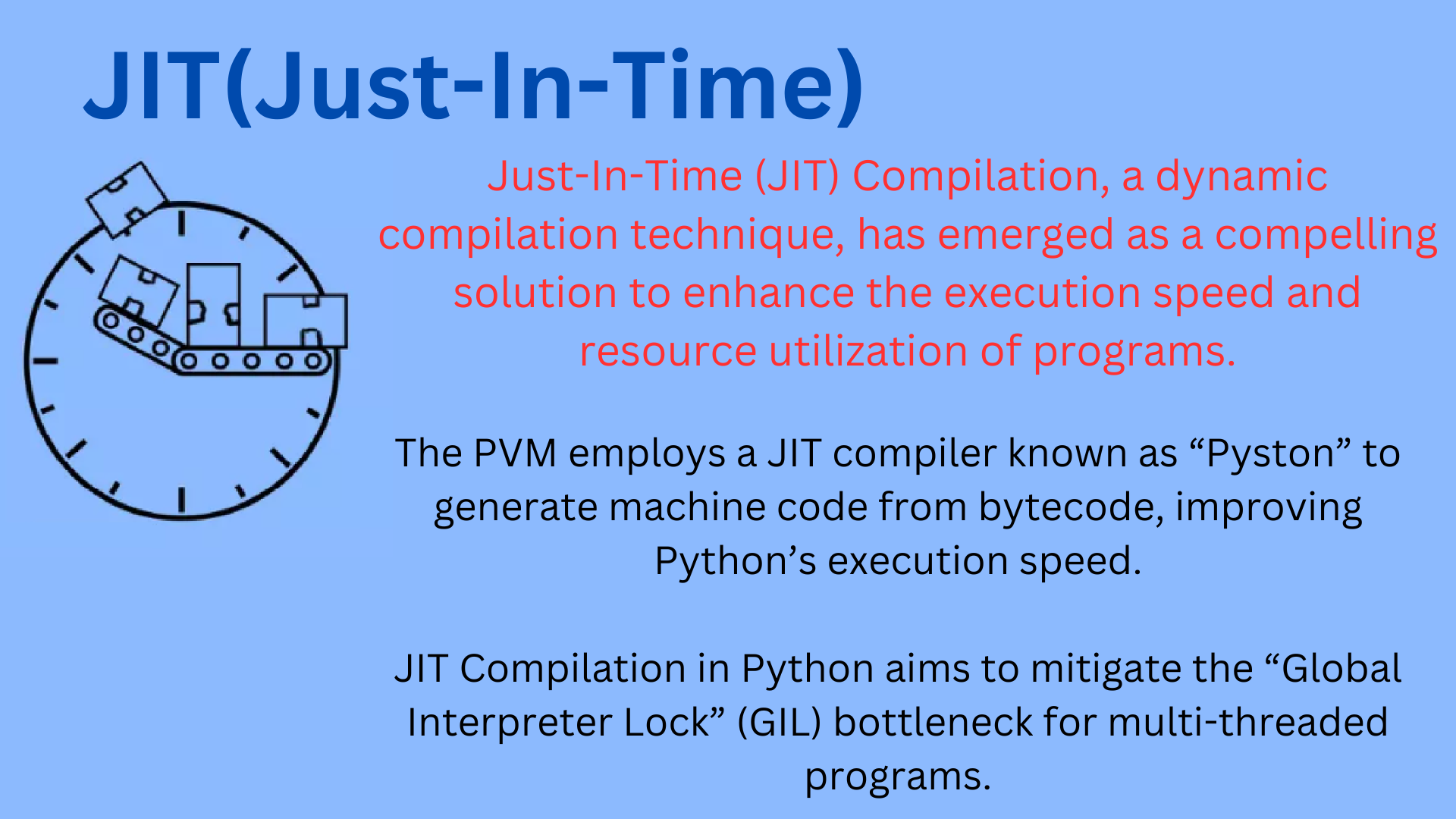
7.Code generator:
This is the final phase of the compiler in which target code for a particular machine is generated. It performs operations like memory management, Register assignment, and machine-specific optimization.
i)Dynamic Typing and Memory Management:
Python is dynamically typed, which means that variable types are determined at runtime. The interpreter manages memory automatically through a mechanism called garbage collection, freeing up memory that is no longer in use.
ii)Exception Handling:
Python has a robust exception handling mechanism that allows you to catch and handle errors gracefully, preventing the program from crashing unexpectedly.
NOTE:
What is Bytecode file? why it is called a Bytecode file?
So, the answer is Bytecode is just like Shorthand language that stores each keyword of java as a sign. and each sign takes 1 byte of memory in RAM. Hence the name called Bytecode file.
Is .pyc file (compiled bytecode) is platform independent?
Compiled Python bytecode files are architecture-independent, but VM-dependent. A .pyc file will only work on a specific set of Python versions .
Conclusion: is Python compiled? Yes. Is Python interpreted? Yes. Sorry, the world is complicated… Python as a programming language has no saying about if it’s an compiled or interpreted programming language, only the implementation of it. The terms interpreted or compiled is not a property of the language but a property of the implementation. Python program runs directly from the source code . so, Python will fall under byte code interpreted. The .py source code is first compiled to byte code as .pyc. This byte code can be interpreted (official CPython), or JIT compiled (PyPy).