Types of Python Operators
- Arithmetic Operators (+, -, /, , %, //, **)
- Assignment Operators (=, +=, -=, /=, =, %=, //=, **=)
- Comparison Operators (==, >, <. >=, <=, !=)
- Logical Operators (and, or, not)
- Membership Operators (in, not in)
- Identity Operators (is, is not)
- Bitwise Operators (|, &, ^, ~, <<, >>)
Arithmetic Operators
Used to perform mathematical operations like addition, subtraction, division, multiplication, modulo, exponent etc..
Operator | Operation | Example |
---|---|---|
+ | Addition | 10 + 2 = 12 |
- | Subtraction | 10 - 2 = 8 |
/ | Division | 10 / 2 = 5.0 |
* | Multiplication | 10 * 2 = 20 |
% | Modulo (Returns Remainder) | 10 % 2 = 0 |
// | Floor Division | 10 // 2 = 5 |
** | Power (a to the power b) | 10 ** 2 = 100 |
Example of Arithmetic Operators
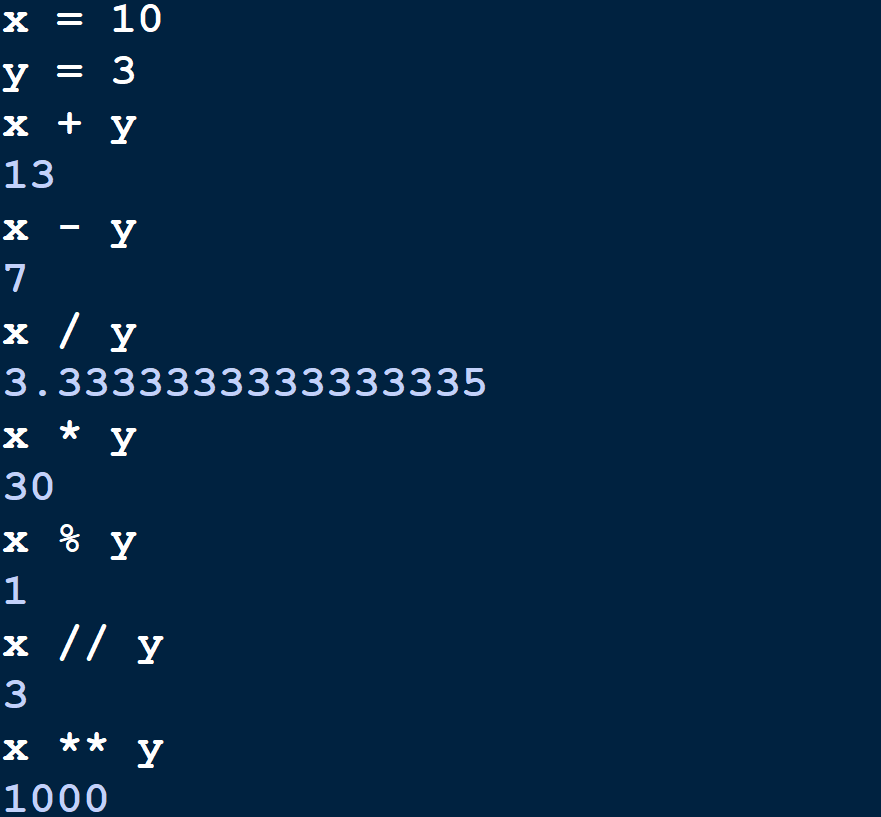
Assignment Operators
Used to assign values to variable.
Operator | Operation | Example |
---|---|---|
= | Assignment | x = 10 |
+= | Addition Assignment | x += 10 (x = x + 10) |
-= | Subtraction Assignment | x -= 10 (x = x - 10) |
/= | Division Assignment | x /= 10 (x = x / 10) |
*= | Multiplication Assignment | x *= 10 (x = x * 10) |
%= | Modulo Assignment | x %= 10 (x = x % 10) |
//= | Floor Division Assignment | x //= 10 (x = x // 10) |
**= | Exponent Assignment | x **= 10 (x = x ** 10) |
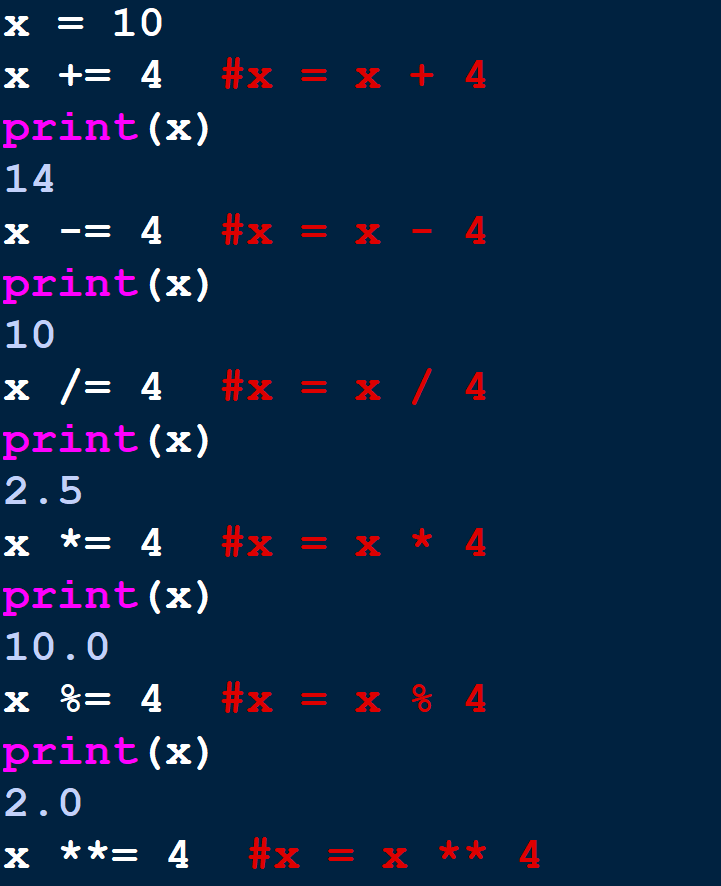
Comparison Operator
Used to compare values of two or more variables and returns true or false.
Operator | Operation | Example |
---|---|---|
== | Is Equal To | 10 == 10 gives True |
!= | Not Equals To | 10 != 10 gives False |
> | Greater Than | 7 > 10 gives False |
< | Less Than | 7 < 10 gives True |
>= | Greater Than or Equal To | 10 >= 10 gives True |
<= | Less Than or Equal To | 12 <= 10 gives False |
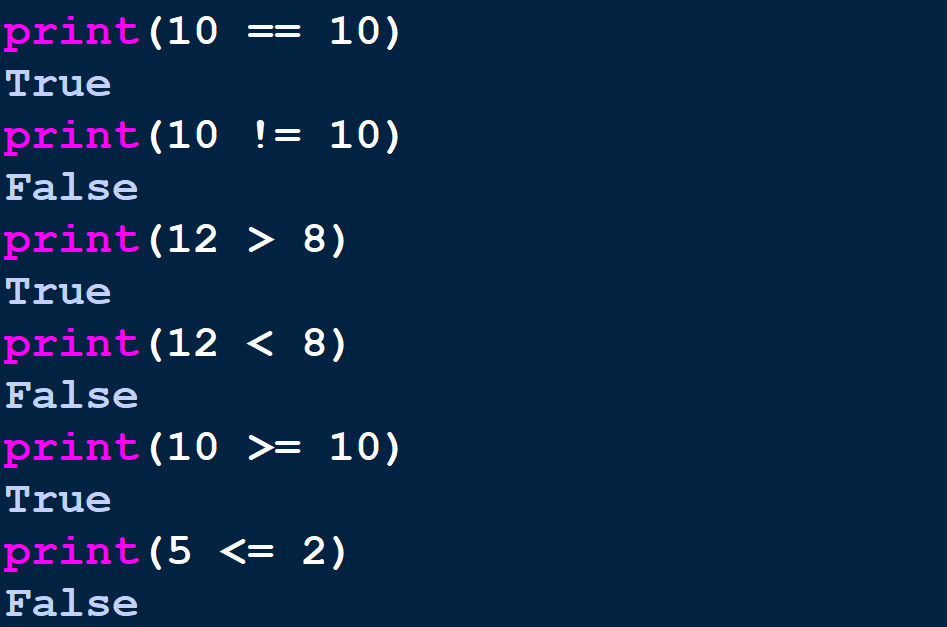
Logical Operators
Used to check whether an expression/condition is True or False. Generally used in decision making.
Operator | Operation | Example |
---|---|---|
and | Logical AND True only if both operands are True |
a and b |
or | Logical OR True if one of the operands is True |
a or b |
not | Logical NOT True if operand if False and vice-versa |
not a |

Membership Operators
Used to test whether a value or a variables is present in a list, tuple, dictionary, set or string.
Operator | Operation | Example |
---|---|---|
in | True if value found in the sequence | "H" in "Hello" gives True |
not in | True if value not found in the sequence | "H" not in "Hello" gives False |

Identity Operators
Used to test whether two objects are identical. It simply checks whether two objects referes to same object in memory.
Operator | Operation | Example |
---|---|---|
is | True if operands are identical (refers to the same object) |
10 is 10 |
is not | True if operands are not identical | 10 is not 10 |

== vs is
Generally asked in a lot of interviews that what exactly is difference between == and is.
== is the comparison operator used to check value of the variables that if both variables contains same value.
is opertor is used to check whether both variables refers to the same address in the memory
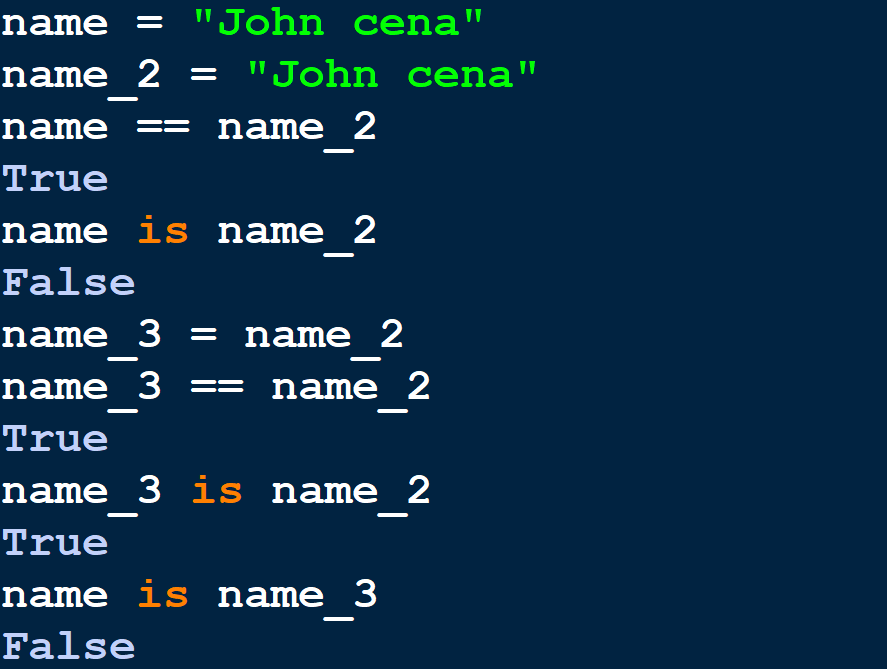
Bitwise Operators
Used to perform operations on binary digits. They convert the number into binary digit and perform binary operation based on whatever operator we are using.
Operator | Operation | Example |
---|---|---|
& | Bitwise AND | x & y |
| | Bitwise OR | x | y |
~ | Bitwise NOT | ~x |
^ | Bitwise XOR | x ^ y |
>> | Bitwise Right Shift | x >> 2 |
<< | Bitwise Left Shift | x << 2 |
Bitwise OR - returns 1 if at least one of the operand is 1
a b a | b
0 0 0
0 1 1
1 0 1
1 1 1
Bitwise AND - returns 1 if both the operands are 1
a b a | b
0 0 0
0 1 0
1 0 0
1 1 1
Bitwise XOR - returns 1 if and only if one of the operand is 1
a b a | b
0 0 0
0 1 1
1 0 1
1 1 0
Bitwise Complement - convert the binary digit from 0 to 1 and vice versa
1s Complement : changes 0 to 1 and 1 to 0
2s Complement : first find 1s Complement then add 1
Left Shift Operator - shifts the bits to the left by specified number of bits
if x << y => x * (2 ** y)
Right Shift Operator - shifts the bits to the right by specified number of bits
if x >> y => x / (2 ** y)
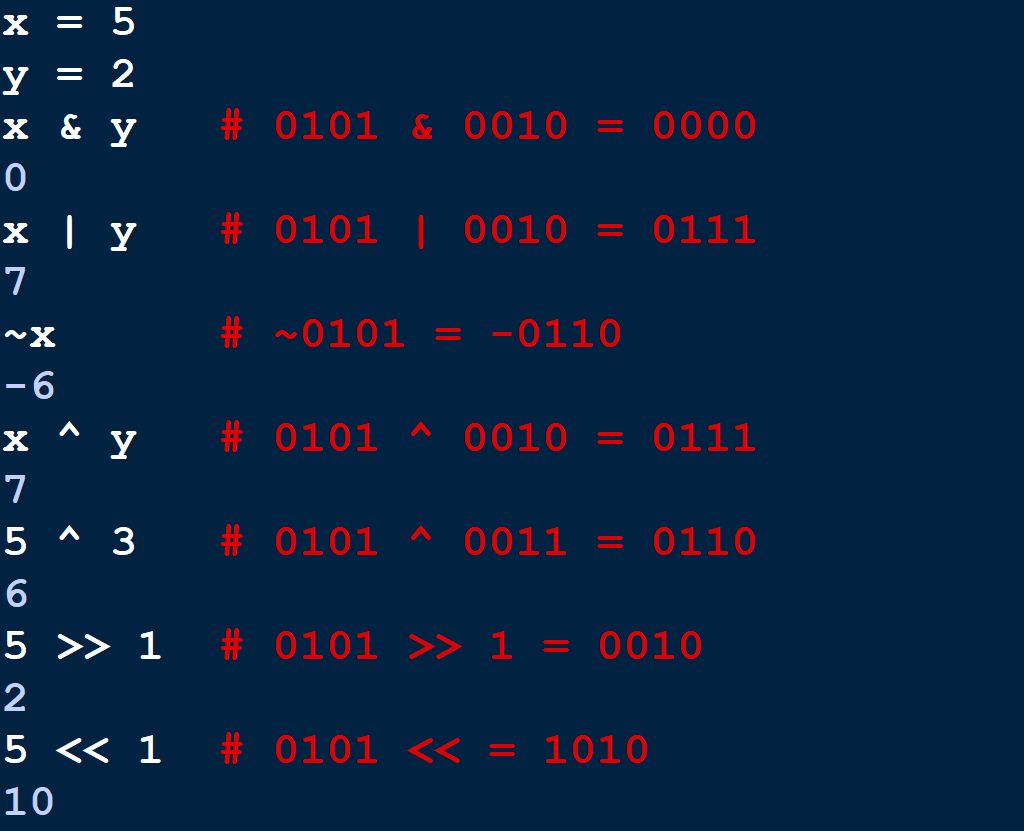
That's all for the Python Operators..