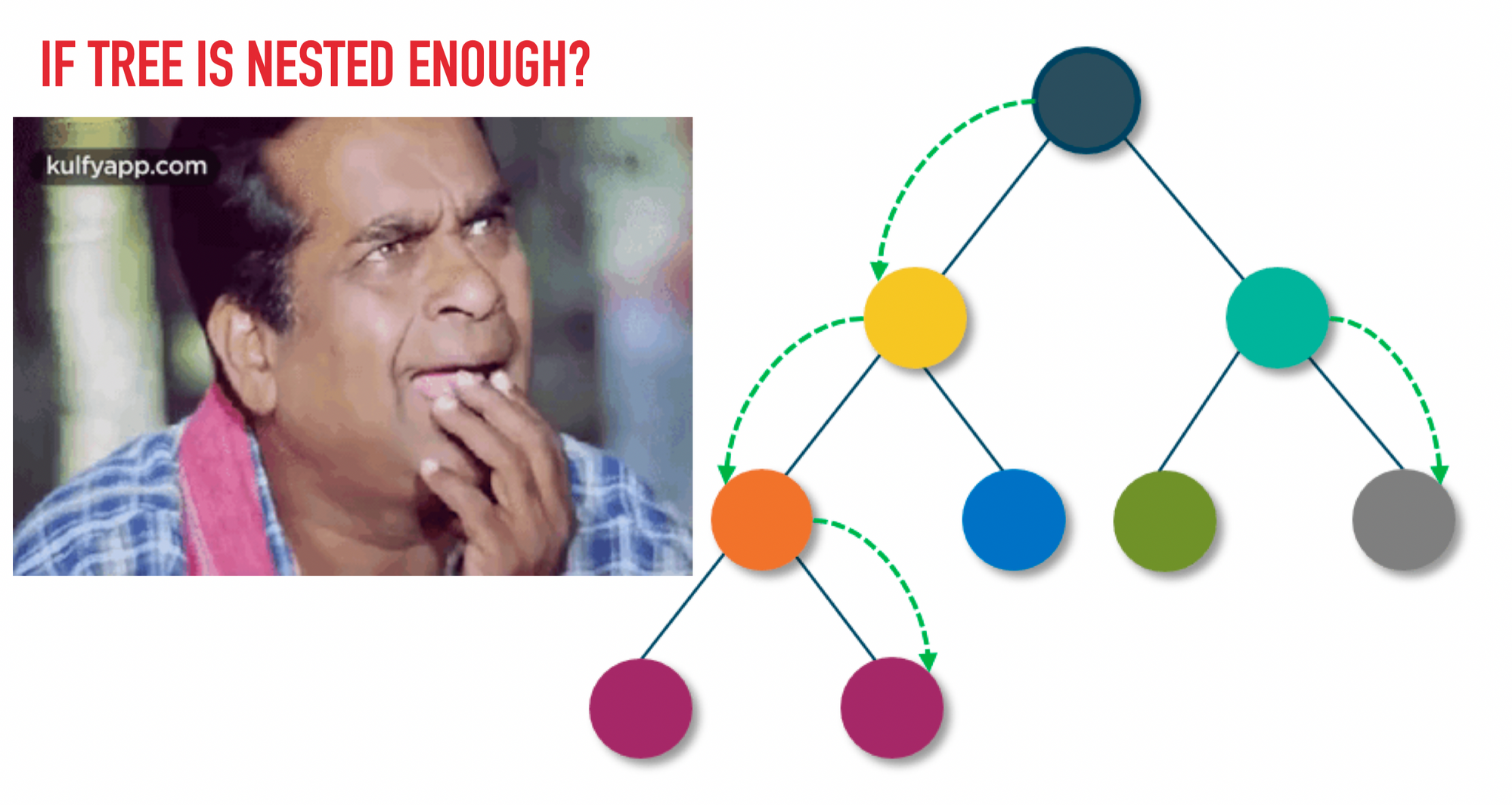
React context is an important thing for every React developer. It lets you easily share state in your applications.
This is the alternative to "prop drilling" or moving props from grandparent to child to parent, and so on. Context is also touted as an easier, lighter approach to state management using Redux.
React context allows us to pass down and use (consume) data in whatever component we need in our React app without using props.
In other words, React context allows us to share data (state) across our components in more easy manner.
Steps to use Context API
Step-1 Create Context
export const UserContext = React.createContext();
Note : React.createContext() is all you need. It returns a consumer and a provider.
Step-2 Provide the Context.
export default function App() {
return (
<UserContext.Provider value="Reed">
<User />
</UserContext.Provider>
)
}
Note: Provider is a component that as it's names suggests provides the state to its children. It will hold the "store" and be the parent of all the components that might need that store.
Step-3 Consume the Context
function User() {
return (
<UserContext.Consumer>
{value => <h1>{value}</h1>}
{/* prints: Reed */}
</UserContext.Consumer>
)
}
Note: Consumer as it so happens is a component that consumes and uses the state.
Or you can consume by Using a Hook. From React 16.8 useContext Hook (to consume the context)
function User() {
const value = React.useContext(UserContext);
return <h1>{value}</h1>;
}
That's all you need.
Let's see in an example. Making 2 Components Login and Register. Register share the user info to the Login component via Context API
Create a Context
import React from 'react';
export const UserContext = React.createContext({user:{},setUser:()=>null});
Here setUser call by Register component and user object is consume by Login.
import React, { useContext } from 'react'
import { useRef } from 'react'
import { UserContext } from '../app/user-context';
import { useState } from 'react';
const Register = () => {
const userid = useRef();
const password = useRef();
const name = useRef();
const context = useContext(UserContext);
const [message, setMessage] = useState('');
const doRegister = ()=>{
const userIdValue = userid.current.value;
const passwordValue = userid.current.value;
const nameValue = userid.current.value;
context.setUser({'userid':userIdValue, 'password':passwordValue, 'name':nameValue});
setMessage('Register SuccessFully')
}
return (
<>
<form onSubmit={handleSubmit(onSubmit)}>
<h1 className='text-center alert-info'>Register</h1>
<p className='alert-info'>{message}</p>
<div className='form-group'>
<label htmlFor="">Userid</label>
<input ref={userid} type="text" className='form-control' placeholder='Type Userid Here' />
</div>
<div className='form-group'>
<label htmlFor="">Password</label>
<input ref={password} type="password" className='form-control' placeholder='Type Password Here' />
</div>
<div className='form-group'>
<label htmlFor="">Name</label>
<input ref={name} type="text" className='form-control' placeholder='Type Name Here' />
</div>
<div className='form-group'>
<button className='btn btn-primary' onClick={doRegister}>Register</button>
</div>
</form>
</>
)
}
export default Register
Consuming in Login.jsx
import React, { useContext } from 'react'
import { useRef } from 'react'
import { UserContext } from '../app/user-context';
const Login = () => {
const userid = useRef();
const password = useRef();
const name = useRef();
const context = useContext(UserContext);
const doLogin = async ()=>{
const userIdValue = userid.current.value;
const passwordValue = userid.current.value;
}
return (
<>
<p>Welcome {context?.user?.name}</p>
<h1 className='text-center alert-info'>Login</h1>
<div className='form-group'>
<label htmlFor="">Userid</label>
<input ref = {userid} type="text" className='form-control' placeholder='Type Userid Here' />
</div>
<div className='form-group'>
<label htmlFor="">Password</label>
<input ref={password} type="password" className='form-control' placeholder='Type Password Here' />
</div>
<div className='form-group'>
<button className='btn btn-primary' onClick={doLogin}>Login</button>
</div>
</>
)
}
export default Login
Home.jsx Providing the Context
<UserContext.Provider value={{user:userInfo,setUser:setUserInfo}}>
{screenFlag?<Login/>:<Register/>}
</UserContext.Provider>
That's all Folks , Happy Learning :)