Introduction
In the vast realm of Java programming, understanding the concept of immutability is crucial for building robust and efficient applications. Immutable objects, once created, cannot be modified, ensuring stability and consistency in your code. One such fundamental concept is the immutable nature of strings in Java. In this blog, we'll delve into the world of immutable strings, exploring what makes them immutable, their benefits, and how to work with them effectively.
What are Immutable Strings?
In Java, strings are immutable, meaning that their value cannot be changed once a string object is created. This immutability is achieved by declaring the String class as final and not providing any methods that modify the contents of the string. Instead, operations on strings create new string objects.
Benefits of Immutable Strings
- Thread Safety: Immutable strings contribute to thread safety in a Java program. Since their values cannot be changed, multiple threads can safely access and share string objects without the risk of data corruption.
- Caching: Java maintains a string pool or string constant pool, where it caches literal strings. When a new string is created, Java first checks if it already exists in the pool. If it does, the existing string is returned, promoting reusability and saving memory.
- Security: Immutable strings enhance security by preventing unintended modification of sensitive data. For example, when dealing with passwords or cryptographic operations, using immutable strings ensures that the original values remain unchanged.
- Hashcode Stability: The immutability of strings guarantees that their hashcode remains constant throughout their lifecycle. This property is essential when using strings as keys in hash-based data structures like HashMap.
How Strings Achieve Immutability
- Final Class: The String class in Java is declared as final, meaning it cannot be extended. This prevents any subclass from introducing mutability.
- Final Fields: The internal representation of strings uses private final char arrays to store their values. Once a string is created, its array of characters is fixed, ensuring the immutability of the string.
- No Setter Methods: The String class provides no methods to modify its content. Operations like concat(), replace() or substring() create new string objects with the desired modifications.
Storing Strings in the String Pool
Java maintains a special pool called the "String Pool" or "String Constant Pool," where literal strings are cached to promote reusability. When a string is created using a string literal, Java checks if the string already exists in the pool. If it does, the existing string is returned, avoiding the creation of a new object.
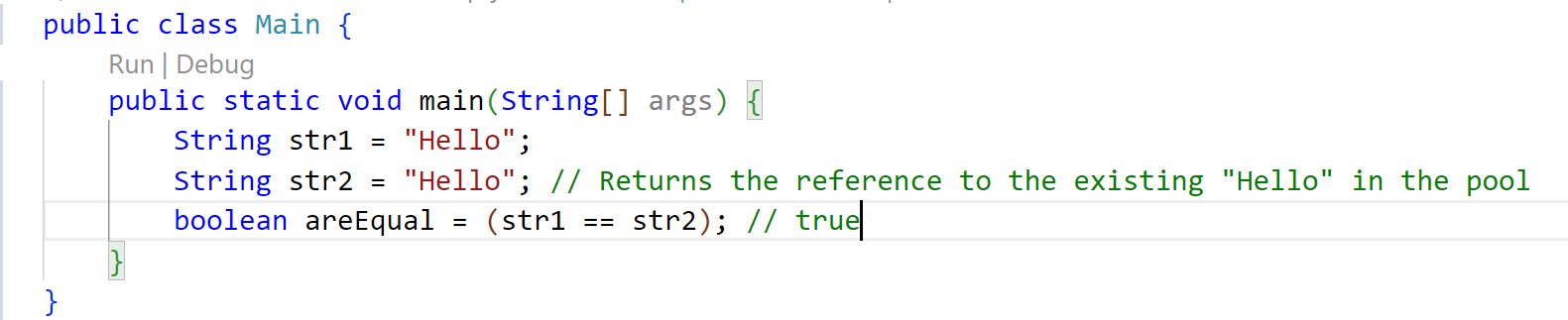
Working with Immutable Strings:
String Concatenation:
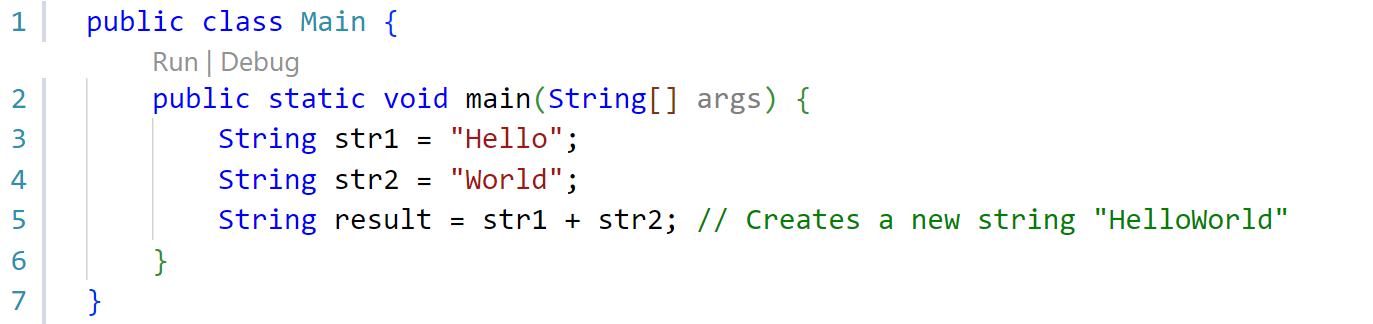
StringBuilder and StringBuffer:
When dealing with extensive string manipulations, consider using StringBuilder or StringBuffer.
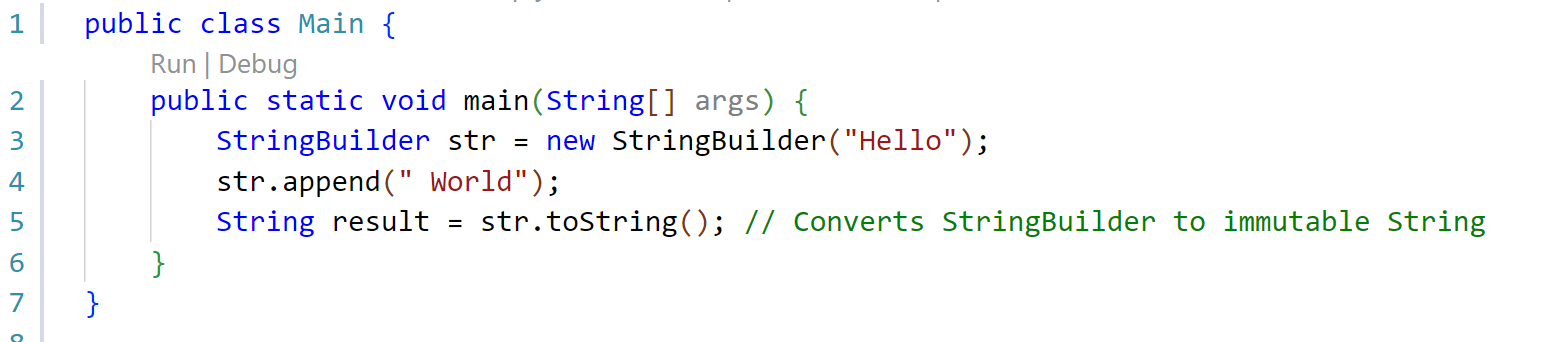
Although these classes are mutable, they are more efficient for concatenating strings repeatedly.
Using Methods:
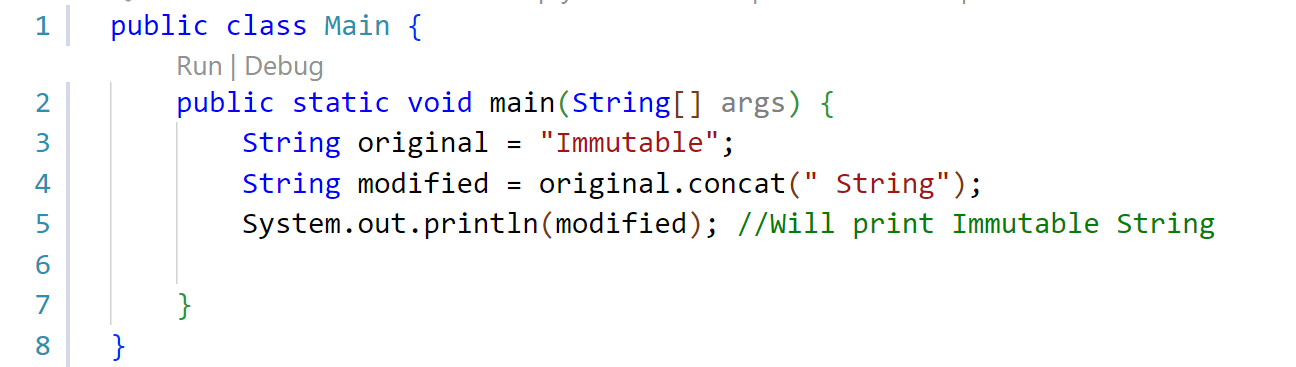
Understanding the concept of immutable strings in Java is essential for writing efficient and reliable code. The inherent thread safety, caching benefits, and security enhancements make immutable strings a cornerstone in Java programming. By embracing immutability, developers can create more predictable and maintainable software, contributing to the overall robustness of their applications.
I hope in this blog, you get a clear idea of immutable strings🙌.