Subarray sum is a contiguous part of an array , this part having the sum of elements which are present in it ,i.e, equals to k(target value).
Example:
Input : arr[] = {8,6,-2,6,3,-4}, k = 10
Output : 3
Explanation: Subarray: arr[1…3]have a sum exactly equal to 10.
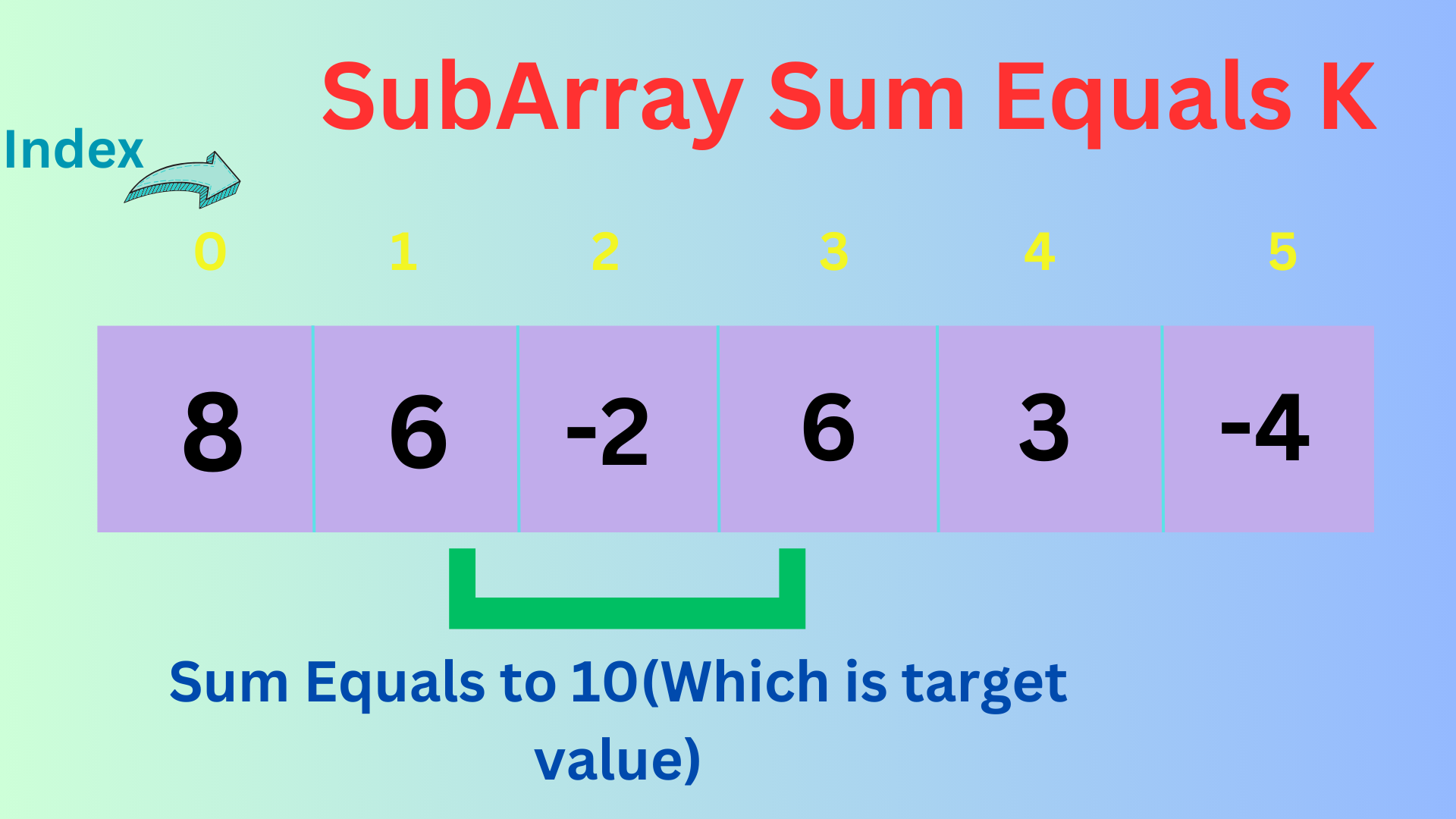
There are following methods to solve this problem :-Using nested For loop , HashMap etc.
Method 1:
Using Nested for loop,there are following steps to implement this approach:
1.Create one variable of name sum to store the sum of arrays element, Sum = 0 and second variable for storing the number of subarrays,count = 0;
2.Now, using nested for loops finding the subarrays which have sum equals to k.
3.If sum equals to k ,then count increased by 1.
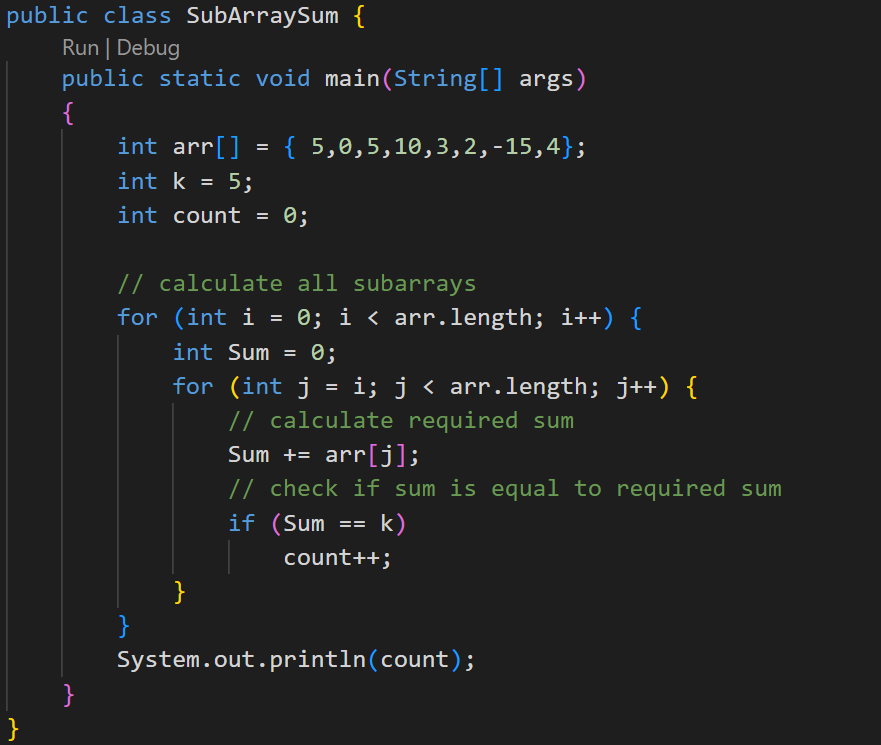
Output:

Time Complexity of this approach is O(n2), where n is the number of elements in the array.
This is the simplest approach , but not the optimized.
Method 2:
Using HashMap technique,there are following steps to implement this approach:
1.Take a hashmap list and put value in it key is "0" and value is "1".
2. Let's take two variables for sum and count ,both are initialized by value "0" and
in map put key is "0" and value is "1".
3.Traverse the array and perform cumulative sum of elements , check if map.containskey(sum-k),then count update.
4.Otherwise , put sum as key in hashMap and put its value by 1 and in the end count is return by the function.
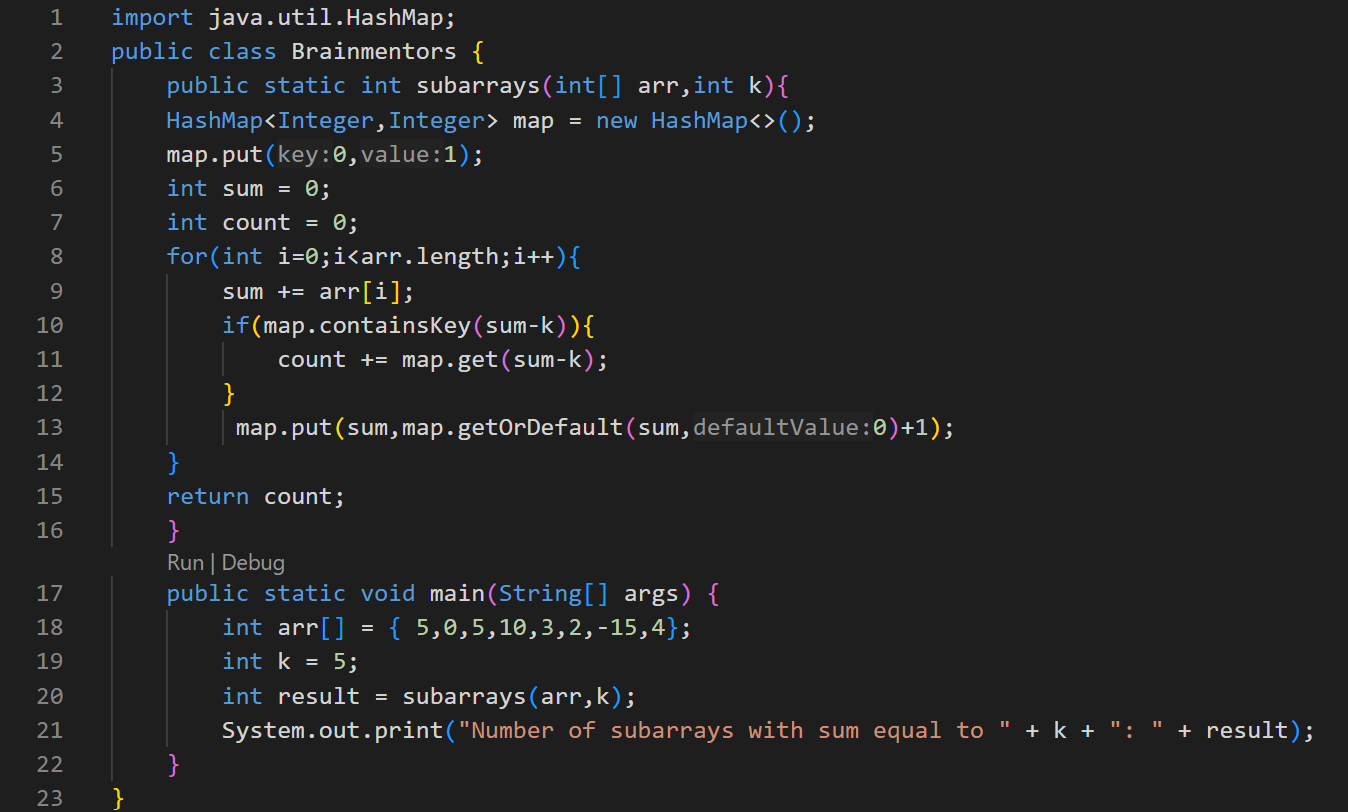
Output:

Note:-Time Complexity of this approach is O(n), where n is the number of elements in the array.