Q1. Problem Statement
Implement a program to find out whether a number is divisible by the sum of its digits.
Display appropriate messages.
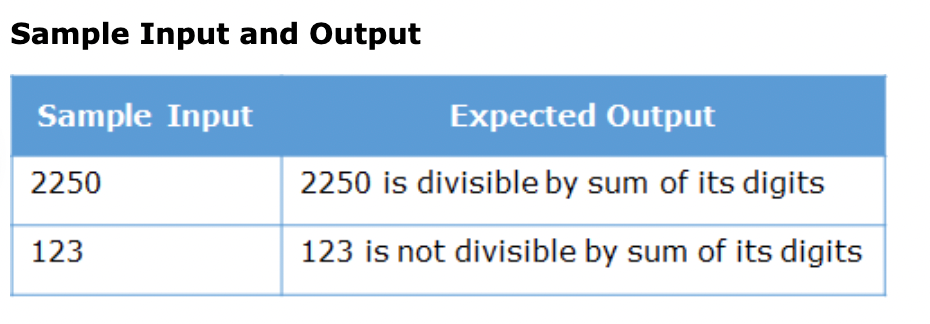
Q2. Implement a program to find out whether a number is a seed of another number.
A number X is said to be a seed of number Y if multiplying X by its every digit equates to Y.
E.g.: 123 is a seed of 738 as 123*1*2*3 = 738
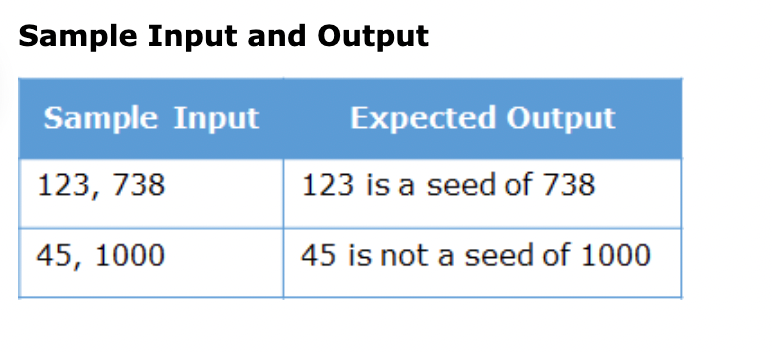
Q3. Problem Statement
Implement a program to check whether a given number is a lucky number.
A lucky number is a number whose sum of squares of every even-positioned digit (starting from the second position) is a multiple of 9.
E.g. - 1623 = 62+32 = 45 is a multiple of 9 and hence is a lucky number.
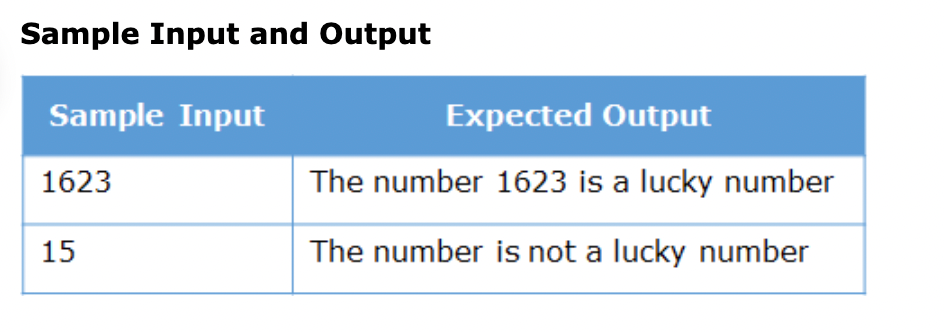
Q4. Write a program to calculate the sum of following series where n is input by user.
1 + 1/2 + 1/3 + 1/4 + 1/5 +…………1/n
Q5. Print the Multiplication Table from 1 to N
1x1 = 1, 2x1 = 2, 3x1 = 3, 4x1 = 4, 5x1 = 5, 6x1 = 6, 7x1 = 7, 8x1 = 8
1x10 = 10, 2x10 = 20, 3x10 = 30, 4x10 = 40, 5x10 = 50, 6x10 = 60, 7x10 = 70, 8x10 = 80
Q6. Write a program to find the Armstrong number for a given range of number.
Input : 153
Expected Output :
Yes it is ArmStrong Number
Armstrong number is a number that is equal to the sum of cubes of its digits. For example 0, 1, 153, 370, 371 and 407 are the Armstrong numbers.
Q7. Write a program to calculate the factorial of a given number.
Input the number : 5
Expected Output :
The Factorial of 5 is: 120
Q8. Write a program to check whether a number is a palindrome or not.
Input a number: 121
Expected Output :
121 is a palindrome number.
Q9. Write a program to convert a decimal number into binary.
Input a decimal number: 10
Output : 1010
Q10. Write a program to sum the even place and odd place digit sum and print it.
Input a number: 4568
Output : Odd Place 4 + 6 = 10
Even Place 5 + 8 = 13
! Enjoy the Questions Happy Coding 😎