
FlutterFireCLI is an Automatic Way . The FlutterFire CLI is a useful tool which provides commands to help ease the installation process of FlutterFire across all supported platforms.
FlutterFire is a set of Flutter plugins which connect your Flutter application to Firebase.
Step-1 Add the firebase_core dependency in pubspec.yaml file
firebase_core: ^2.12.0
Step-2 Initialise FlutterFire
FlutterFire now supports initialisation directly from Dart
Note : Before any of the Firebase services can be used, FlutterFire needs to be initialized (you can think of this process as FlutterFire "bootstrapping" itself). The initialization step is asynchronous, meaning you'll need to prevent any FlutterFire related usage until the initialization is completed.
The FlutterFire CLI depends on the Firebase CLI.
Visit https://firebase.google.com/docs/cli#install-cli-mac-linux
The FlutterFire CLI is a useful tool which provides commands to help ease the installation process of FlutterFire across all supported platforms.
After Installation do firebase login command on terminal
Test that the CLI is properly installed and accessing your account by listing your Firebase projects. Run the following command:
firebase projects:list
To initialize FlutterFire, call the initializeApp method on the Firebase class. The method accepts your Firebase project application configuration, which can be obtained for all supported platforms by using the FlutterFire CLI:
Install the CLI if not already done so
dart pub global activate flutterfire_cli
Run the configure
command, select a Firebase project and platforms
flutterfire configure
Once configured, a firebase_options.dart file will be generated for you containing all the options required for initialization.
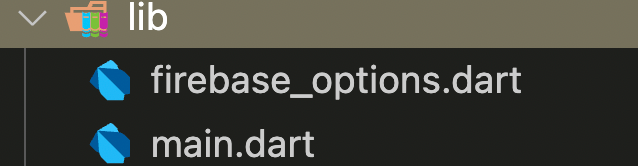
import 'package:flutter/material.dart';
import 'package:firebase_core/firebase_core.dart';
import 'firebase_options.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp(
options: DefaultFirebaseOptions.currentPlatform,
);
runApp(MyApp());
}
FireBase Authentication
Firebase Authentication provides backend services & easy-to-use SDKs to authenticate users to your app. It supports authentication using passwords, phone numbers, popular federated identity providers like Google, Facebook and Twitter, and more.
flutter pub add firebase_auth
For OAuth with Google google_sign_in: ^6.1.0
//Google SignIn Code
import 'package:firebase_auth/firebase_auth.dart';
import 'package:google_sign_in/google_sign_in.dart';
Future<UserCredential> signInWithGoogle() async {
// Trigger the authentication flow
final GoogleSignInAccount? googleUser = await GoogleSignIn().signIn();
// Obtain the auth details from the request
final GoogleSignInAuthentication? googleAuth =
await googleUser?.authentication;
// Create a new credential
final credential = GoogleAuthProvider.credential(
accessToken: googleAuth?.accessToken,
idToken: googleAuth?.idToken,
);
// Once signed in, return the UserCredential
return await FirebaseAuth.instance.signInWithCredential(credential);
}
Add Some Additional Settings
Add SHA Key FingerPrint in Android (FireBase Android Project)

For FingerPrint Generation
https://developers.google.com/android/guides/client-auth?sjid=2646288098421978587-AP
Add Some More Additional Settings
Add these things
Open the Firebase console and click on the settings icon.
You will see the list of the apps connected to your Firebase project
For Android
1. Open terminal inside your flutter project
2. cd android
3. ./gradlew signingReport or gradlew signingReport
4. Paste your SHA1 key
5. Download and replace the google-services.json
6. flutter clean
IOS:
1. Configure your Firebase project
2. Select IOS
3. Enter your Bundle ID
4. Download credetials
5. Download and replace GoogleService-info.plist
Add this to your info.plist
<key>CFBundleURLTypes</key>
<array>
<dict>
<key>CFBundleTypeRole</key>
<string>Editor</string>
<key>CFBundleURLSchemes</key>
<array>
<string>**INSERT HERE YOUR RESERVED_CLIENT_ID FROM GoogleService-Info.plist**</string>
</array>
</dict>
</array>
Finally , Enable Google Sign IN (From FireBase Console)

Final Code
import 'package:firebase_auth/firebase_auth.dart';
import 'package:google_sign_in/google_sign_in.dart';
Future<UserCredential> signInWithGoogle() async {
// Trigger the authentication flow
final GoogleSignInAccount? googleUser = await GoogleSignIn().signIn();
// Obtain the auth details from the request
final GoogleSignInAuthentication? googleAuth =
await googleUser?.authentication;
// Create a new credential
final credential = GoogleAuthProvider.credential(
accessToken: googleAuth?.accessToken,
idToken: googleAuth?.idToken,
);
// Once signed in, return the UserCredential
return await FirebaseAuth.instance.signInWithCredential(credential);
}
Login Option
import 'package:flutter/material.dart';
import 'package:flutter/src/widgets/framework.dart';
import 'package:flutter/src/widgets/placeholder.dart';
import 'package:flutter_firebase_example/models/user_data.dart';
import '../services/auth.dart';
class Login extends StatefulWidget {
const Login({super.key});
@override
State<Login> createState() => _LoginState();
}
class _LoginState extends State<Login> {
_doLogin() async {
try {
final response = await signInWithGoogle();
print("Response is $response");
UserData userData = UserData(
displayName: response.user!.displayName!,
photo: response.user!.photoURL!);
Navigator.pushNamed(context, '/crud', arguments: userData);
//print(response.user?.displayName);
} on Exception catch (e) {
print("Google Login Fails :::::: ");
print(e);
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('OAuth Example'),
),
body: Column(
children: [
ElevatedButton(
onPressed: () {
_doLogin();
},
child: Text('Google Login'))
],
),
);
}
}
Connect with FireStore
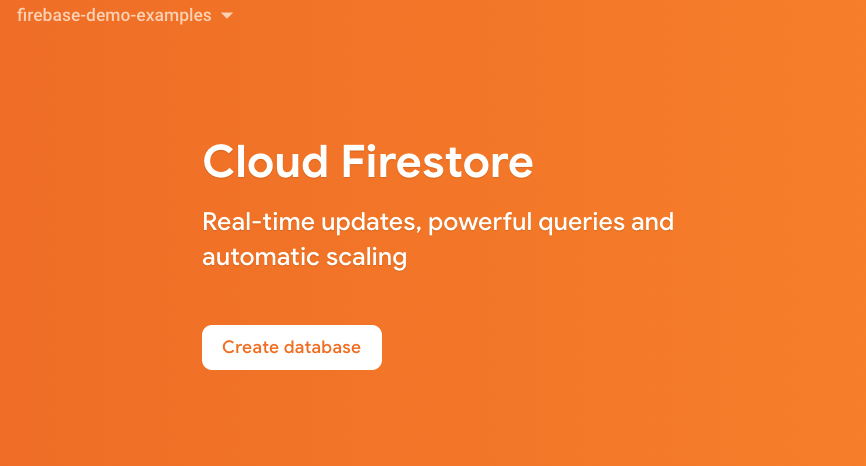
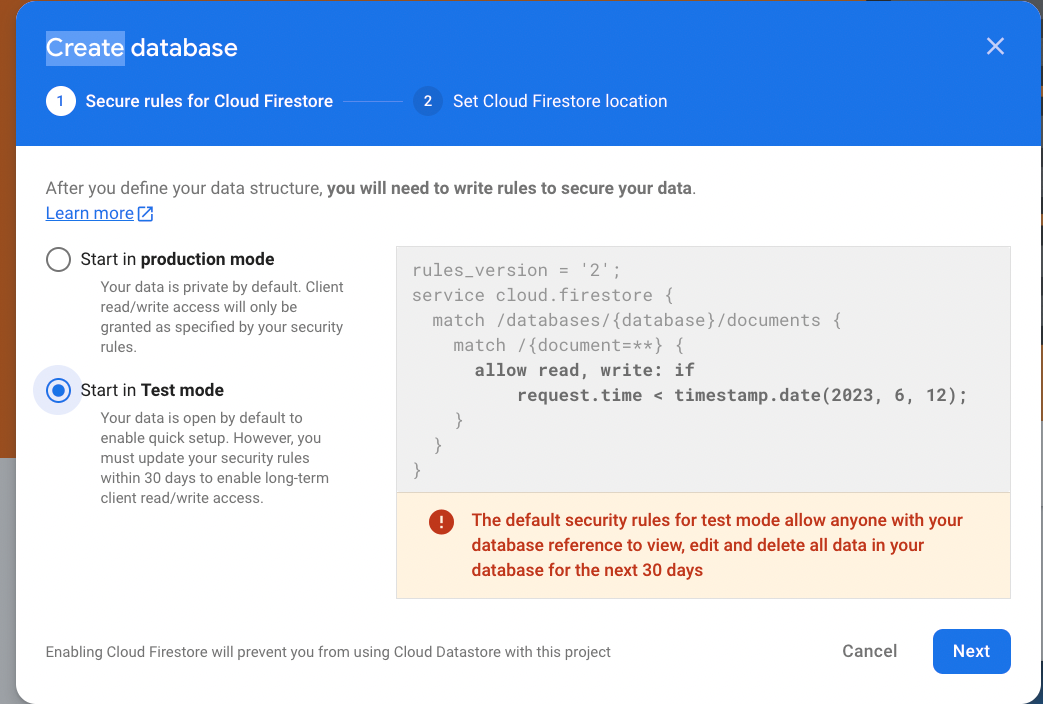
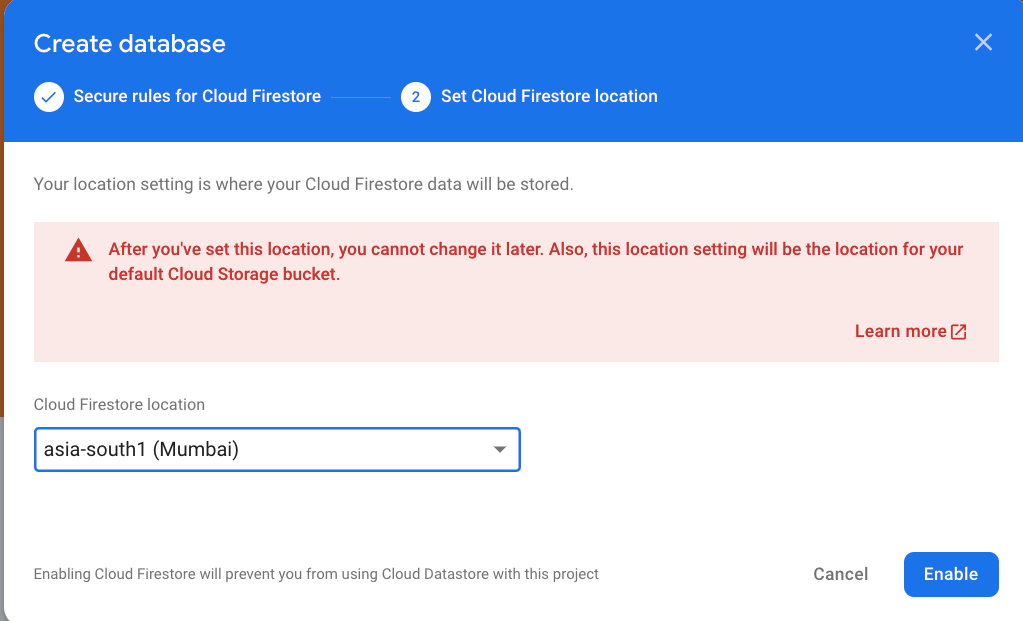
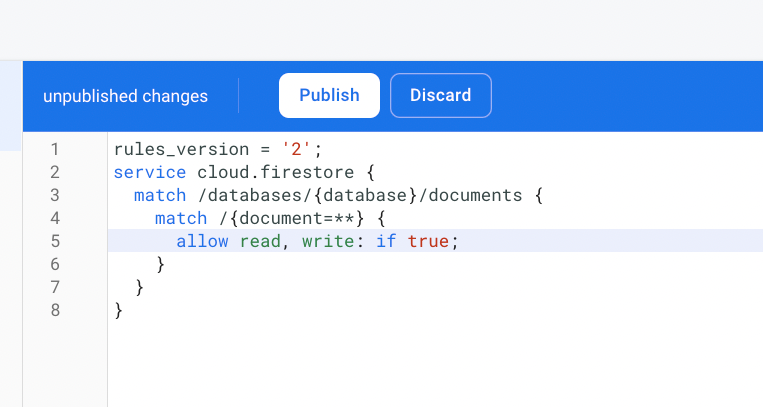
// Install FireStore
//flutter pub add cloud_firestore
import 'package:cloud_firestore/cloud_firestore.dart';
import 'package:firebase_core/firebase_core.dart';
import 'package:flutter_firebase_example/models/user_data.dart';
class CrudService {
Future<DocumentReference> add(UserData userData) async {
return await FirebaseFirestore.instance
.collection('tasks')
.add(userData.toJSON());
}
Stream<QuerySnapshot<Map<String, dynamic>>> read() {
return FirebaseFirestore.instance.collection('tasks').snapshots();
}
}
That's All Folks for this Tutorial , Catch you in next one , Happy Learning :)