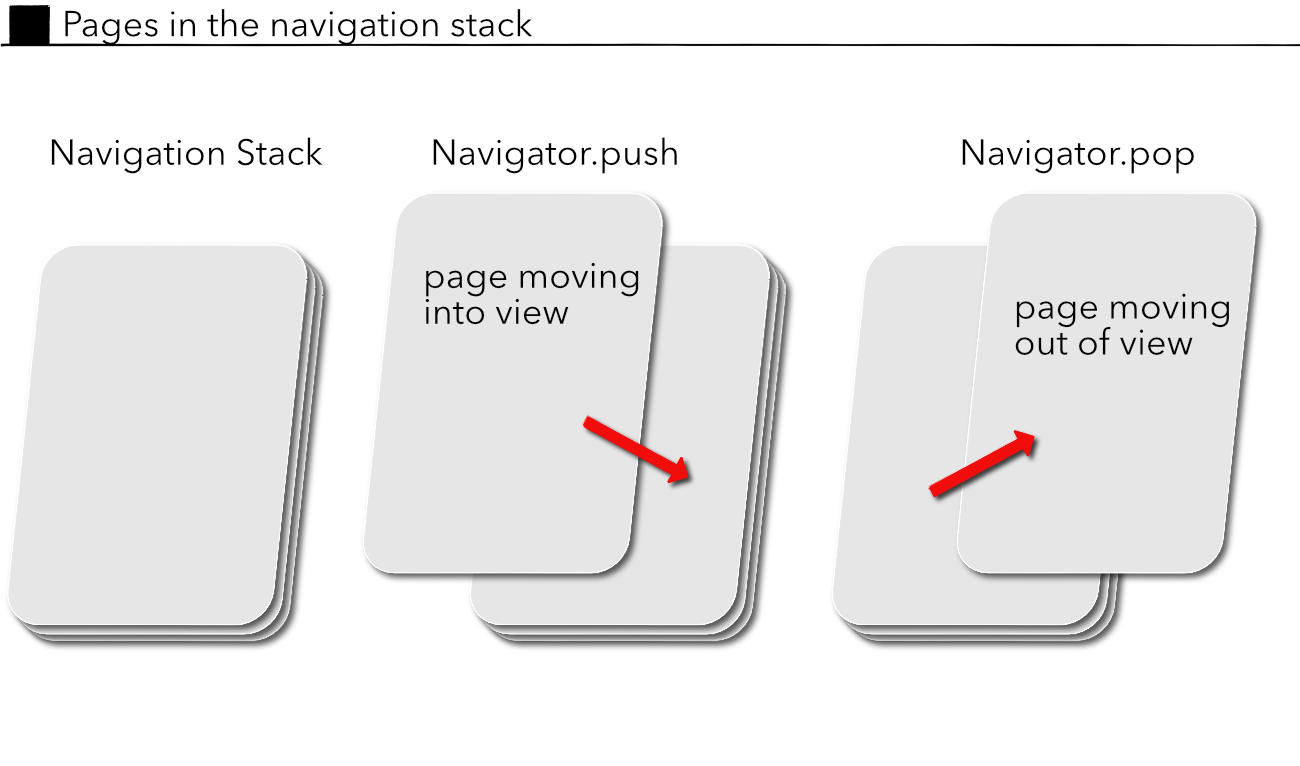
Navigator.push(
context,
MaterialPageRoute(builder: (context) => SecondRoute()),
);
Named Route - if ur application is big, maintain the routes on single place
e.g in the main dart file
Named Routes
MaterialApp(
title: 'Named Routes Demo',
// Start the app with the "/" named route. In this case, the app starts
// on the FirstScreen widget.
initialRoute: '/',
routes: {
// When navigating to the "/" route, build the FirstScreen widget.
'/': (context) => const FirstScreen(),
// When navigating to the "/second" route, build the SecondScreen widget.
'/second': (context) => const SecondScreen(),
},
)
Navigate to Second Screen
// Within the FirstScreen
widget
onPressed: () {
// Navigate to the second screen using a named route.
Navigator.pushNamed(context, '/second');
}
Return to the first screen
// Within the SecondScreen widget
onPressed: () {
// Navigate back to the first screen by popping the current route
// off the stack.
Navigator.pop(context);
}
For handling the arguments
// You can pass any object to the arguments parameter.
// In this example, create a class that contains both
// a customizable title and message.
class ScreenArguments {
final String title;
final String message;
ScreenArguments(this.title, this.message);
}
Extracting the Arguments in the Second Screen
final args = ScreenArguments;
Doing the Navigation from the first Screen
Navigator.pushNamed(
context,
ExtractArgumentsScreen.routeName,
arguments: ScreenArguments(
'Extract Arguments Screen',
'This message is extracted in the build method.',
),
);