Dart is a a truly object-orientated language.
What is Class - It is a Blue Print.
What is an Object - It is an instance of a class.
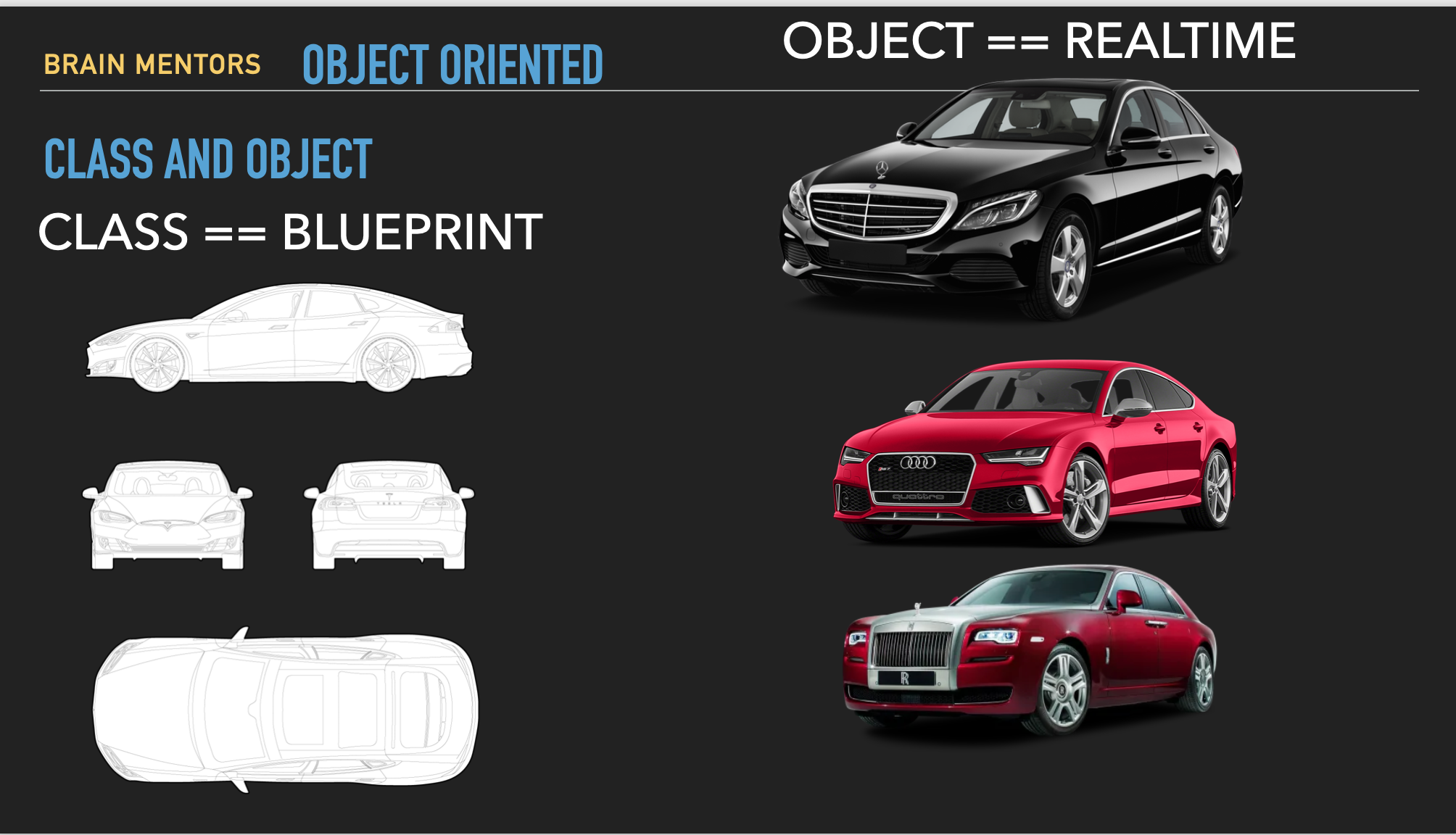
Syntax to build a class.
class Emp{
int id; // default is null
String name;
}
Creating Object
Emp emp = Emp(); // The new keyword became optional in Dart 2.
What is Constructor?
A constructor is a special method of a class that is used for initializing the variables of the class. A constructor does not allow specifying custom return type, the return type of the constructor is the class itself.
If no constructor is defined by the programmer then default constructor is the no-argument constructor.
class A{
A(){
// Default Constructor
}
}
Calling Default Constructor
void main(){
A obj = A();
}
Declare a constructor by creating a function with the same name as its class, Additionally u can define Named Constructors. Also If you have more than one constructor u need Named constructor.
Rules for defining both default and parameterized constructor
the rules for defining both default and parameterized constructors in Dart programming language
- Name of both default and parameterized constructors in Dart is name of the class itself.
- A default constructor does not have a parameter. A Parameterized constructor can have one or more parameters.
- Constructor body: The bode of the constructor is executed whenever a constructor is called during creation of a new object.
- Constructors do not allow defining custom return types, the return type of the constructor is the class itself.
Parameterized Constructor
A constructor that accepts parameters is called parameterized constructor. Similar to functions, constructors can also accept parameters. Parameterized constructors allows a programmer to initialize un-initialized variables with a custom value.
class A{
late int x;
A(int x){
this.x = x;
}
}
void main(){
A obj = A(10);
}
ShortHand Way of Writing constructor
class A{
late int x;
A(this.x);
}
void main(){
A obj = A(10);
}
Named constructors
Use a named constructor to implement multiple constructors for a class or to provide extra clarity:
class Emp{
Emp.myCons(){
}
}
😎 Note : If you don’t declare a constructor, a default constructor is provided for you. The default constructor has no arguments and invokes the no-argument constructor in the superclass.
Constant Constructor In Dart
Use const constructors if you want to create objects that never change and are treated as compile time constants.
A class can have constant constructor only if all of its members are final.
If a class has any non-final field then the class can not have a constant constructor, it simply can not be defined.
class A {
final int x;
final int y;
const A(this.x, this.y);
}
void main(){
A obj1 = const A(100, 200);
A obj2 = const A(100, 200);
A obj3 = const A(100, 200);
print(identical(obj1, obj2));
print(identical(obj1,obj3));
print(obj1==obj2);
print(obj1==obj3);
}
Note : Compile-time constants are canonicalized. That means the no matter how many times you write "const A(0,0)", you only create one object. That may be useful - but not as much as it would seem, since you can just make a const variable to hold the value and use the variable instead.
Access Modifier in Dart.
In Java, we can use public, protected, and private keywords to control the access scope for a property or method. However, Dart doesn't provide that kind of keywords. Instead, you can use _ (underscore) at the start of the name to make a data member of a class becomes private.
In Dart, the privacy is at library level rather than class level. It means other classes and functions in the same library still have the access.
The class has two fields: first (public) and _second (private). (No Protected is present in Dart)
Note: Dart Not Provide any Keyword like private , public , protected.
class B {
String _iamPrivate; // Scope to the file , not outside the file (Library).
// What is Library, Every Dart Application is a Library.
String iamPublic;
}
Note: To Access _private outside the file create getter and setter for this.
class B {
String _iamPrivate; // Scope to the file , not outside the file (Library).
String iamPublic;
String get iamPrivate {
return _iamPrivate;
}
void set iamPrivate(String iamPrivate) {
this._iamPrivate = iamPrivate;
}
}
Getter and Setters
Getters and setters are special methods that provide read and write access to an object’s properties. Recall that each instance variable has an implicit getter, plus a setter if appropriate. You can create additional properties by implementing getters and setters, using the get and set keywords:
Example:
double get right => left + width;
set right(double value) => left = value - width;
That's all folks see you in next Tutorial 😎