Introduction
In the vast world of computer science and algorithms, there exists a fascinating problem known as the Celebrity Problem. The problem revolves around identifying a celebrity in a crowd where everyone knows them, but they know nobody. This intriguing problem finds its resolution through an efficient algorithmic approach involving stacks, providing an excellent opportunity to explore the depths of this data structure.
The Celebrity Problem: A Brief Overview
Imagine a party where a group of people is gathered. Among them, there might be a celebrity – a person who is known by everyone but knows none of them.Formally, if there exists a person 'A' in the crowd such that all other people know 'A,' but 'A' knows nobody, then 'A' is the celebrity we seek. The task is to identify this celebrity by asking the fewest possible questions.
The Naive Approach: O(n²) Complexity
Before delving into the optimized stack-based solution, let's first discuss a naive approach to solving the Celebrity Problem. One straightforward method is to check every pair of persons in the group. If person 'A' knows person 'B,' then 'A' cannot be the celebrity. Similarly, if 'A' is not acquainted with 'B,' then 'B' cannot be the celebrity.
Example Scenario:
Let's consider a scenario with 5 people (A, B, C, D, and E). The matrix representing their relationships is as follows:
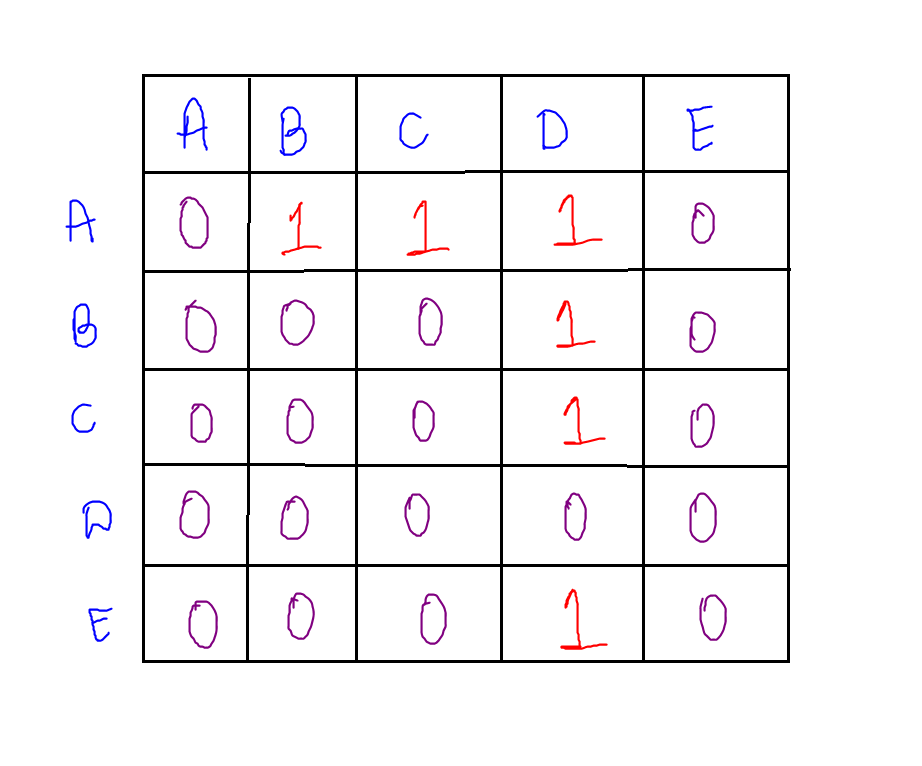
Here, 1 indicates that the corresponding person knows the person of that index, and 0 indicates the absence of such knowledge.
For more ease, we have considered that a person doesn't know himself (represented by a 0)
By iterating through all pairs of individuals, we can potentially identify the celebrity.
Person A!
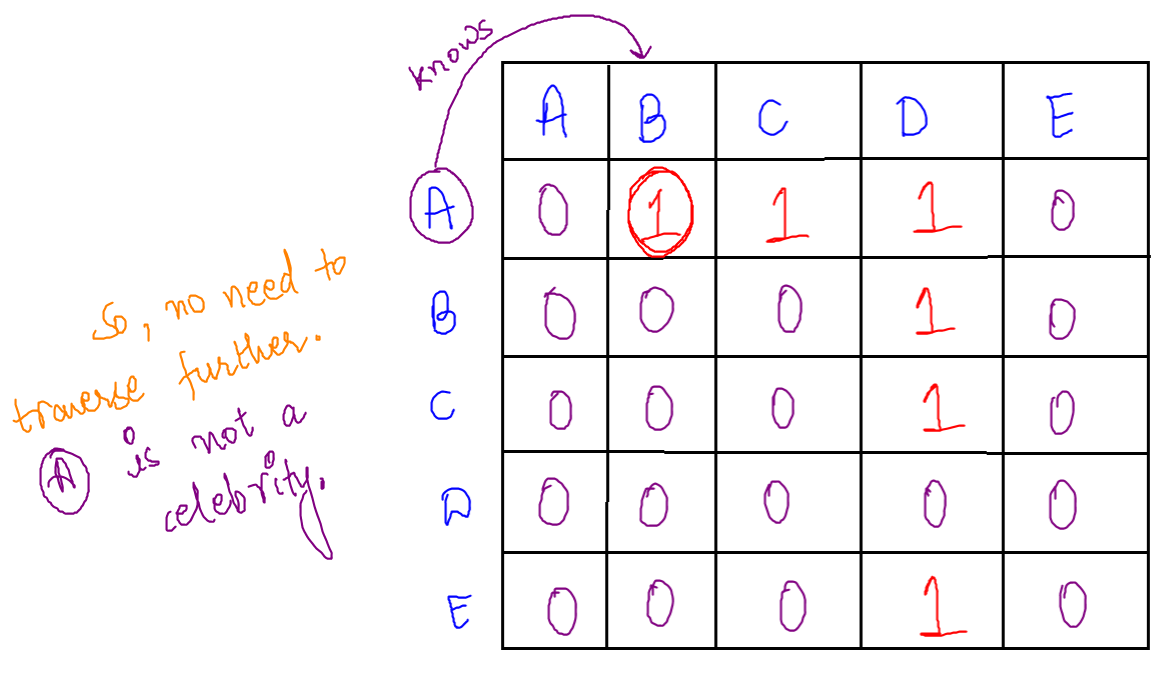
Person B!
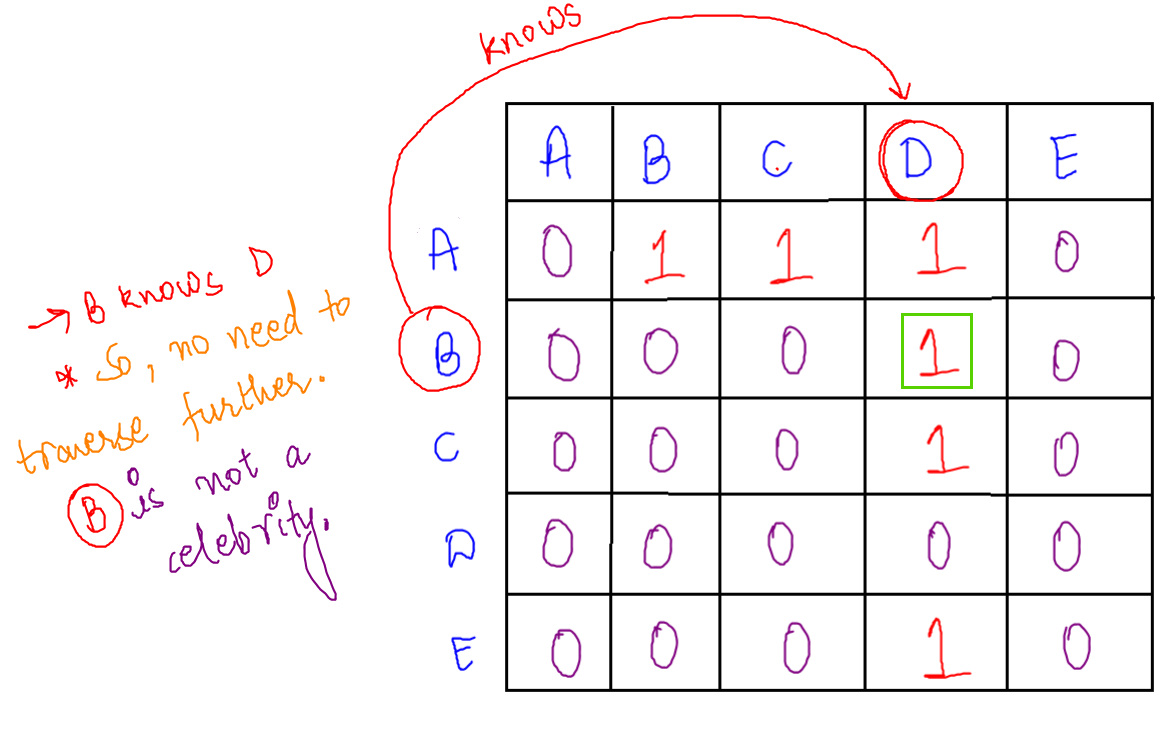
Person C!
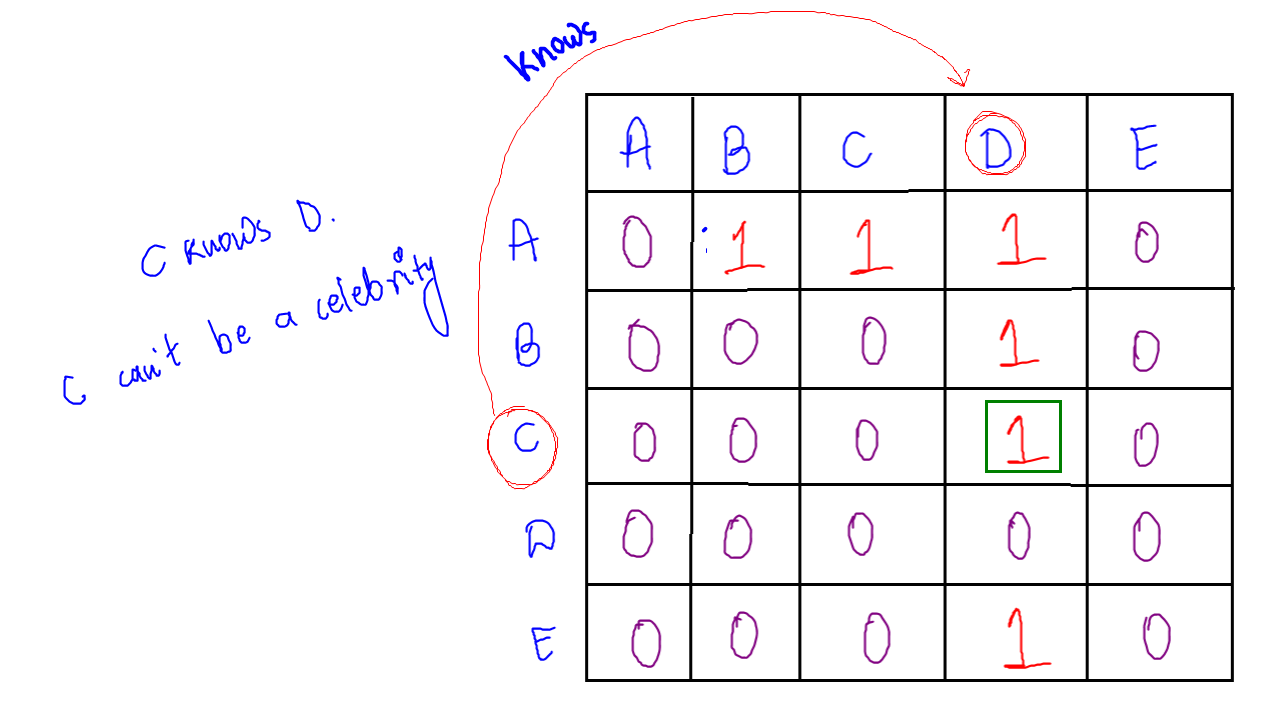
Person D!

Pseudocode for Naive Approach:
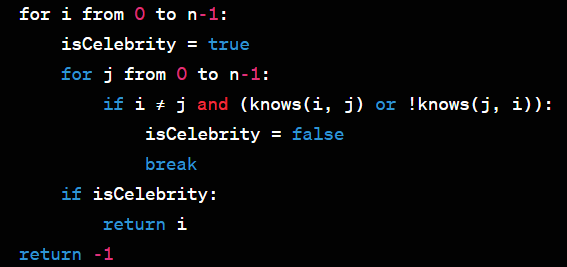
In this naive approach, the outer loop runs 'n' times, and the inner loop also runs 'n' times, making the overall time complexity O(n²), which is inefficient for large datasets.
Solution Using Stacks
The problem can be efficiently solved using stacks. Here’s how:
Using a Stack:
- Push all the people onto a stack.
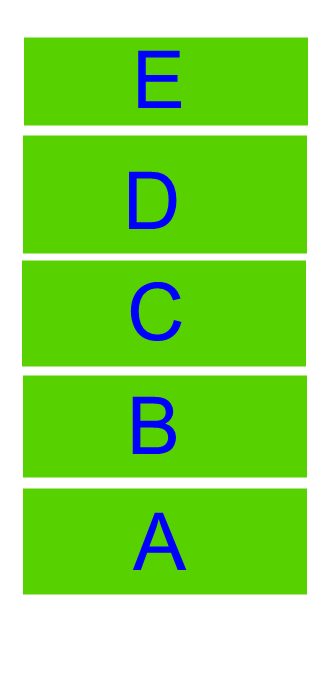
Identifying the Celebrity:
- While there are more than two people in the stack, pop two persons, A and B.
- If A knows B, A cannot be the celebrity. Push B back onto the stack.
- If A doesn't know B, B cannot be the celebrity. Push A back onto the stack.

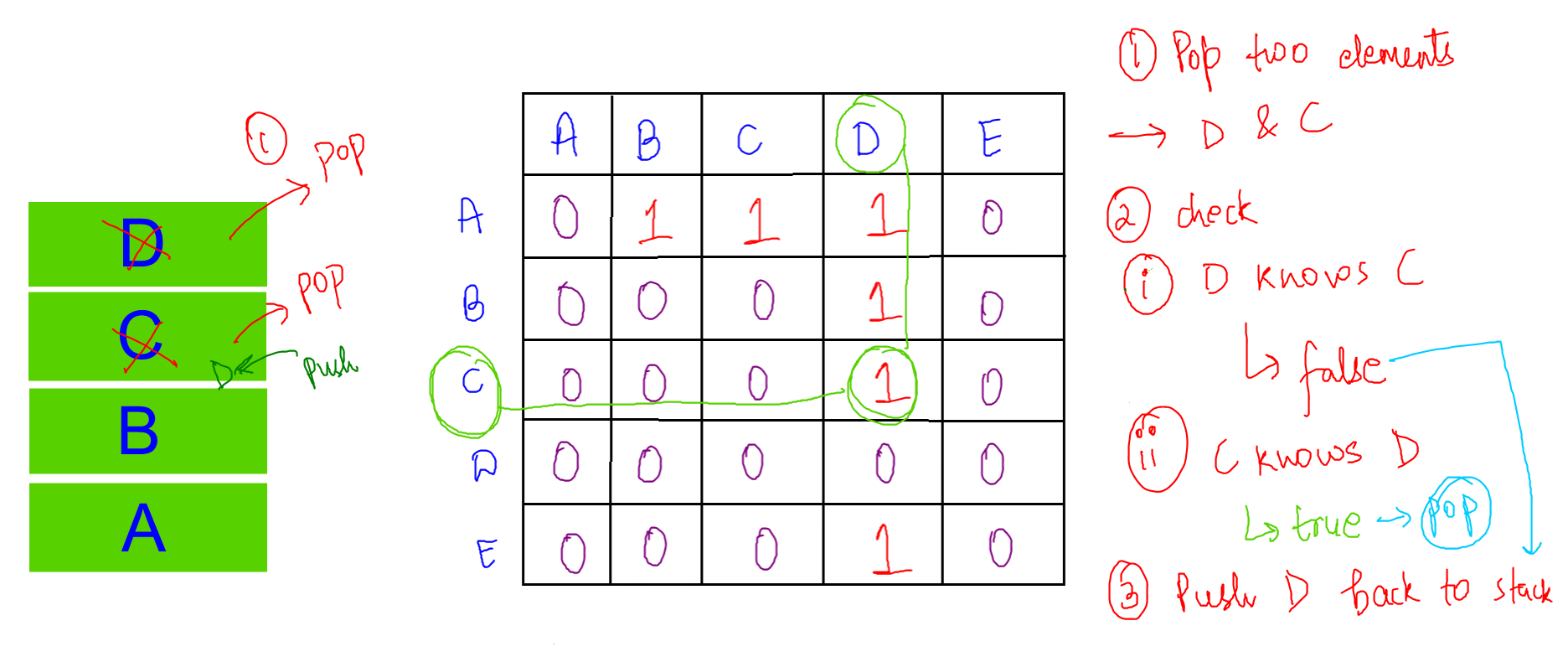
Similarly, u can proceed iteratively further for next elements in the stack
Solution Steps:
- Push all 5 people onto the stack.
- Pop E and D. E knows D, so E is not the celebrity. Push D back.
- Pop D and C. C knows D, so C is not the celebrity. Push D back.
- Pop D and B. B knows D, so B is not the celebrity. Push D back.
- Pop D and A. A knows D, so A is not the celebrity. Push D back.
- Only D remains on the stack. Verify if D is known by everyone and does not know anyone. In this case, D is the celebrity.
The final stack will be like
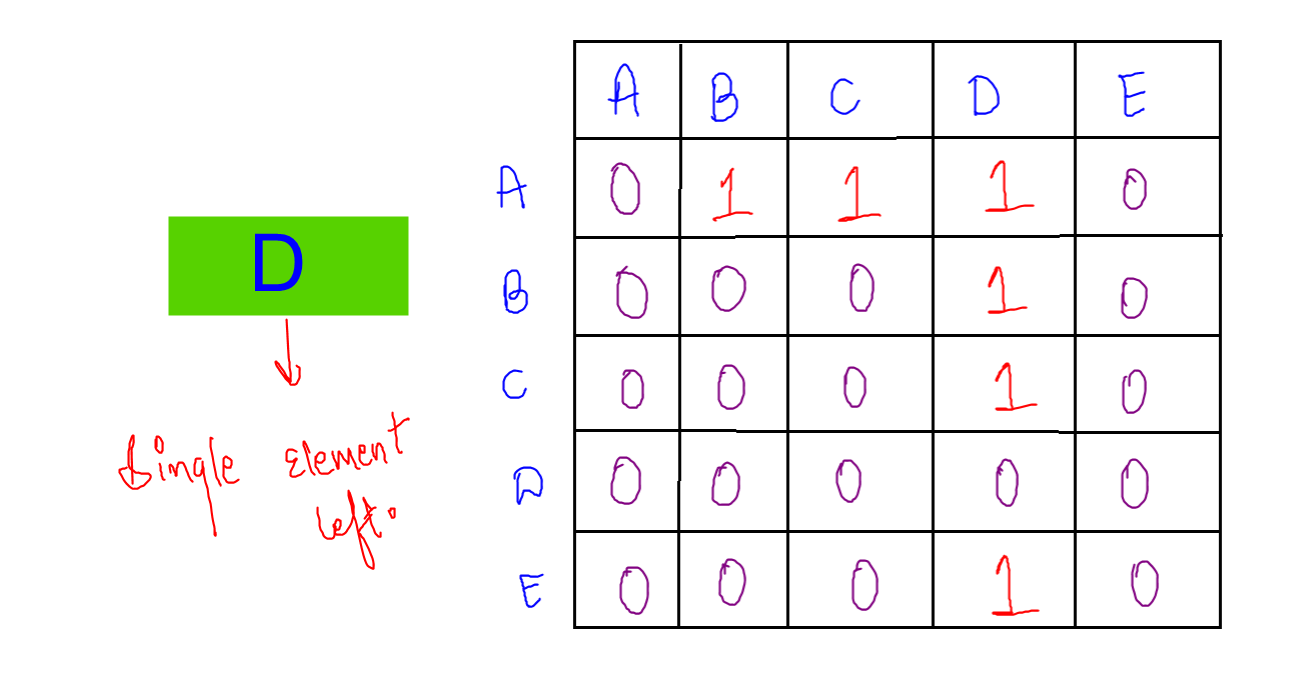
Final Verification:
- The remaining person in the stack (D) is a potential celebrity.
Output:
In this scenario, the algorithm identifies D as the celebrity, providing the desired output.

potentialCelebrity will provide uh the answer!
Hope you would have understand the solution approaches to the problem.
If it's a yes , guys try to code it💻
I hope you all will find this helpful.
Thank you for joining us on this journey. If you found this content helpful and insightful, don't forget to share this article. Stay tuned for more exciting insights, happy learning, and happy trading!"😊😊