Why We need an Array?
To Store a Single Value like price of one product, or marks of one student or salary of one employee we can use variable for this purpose.
e.g double salary ; int marks; double price;
But if you want to store value of 1000 products, marks of 50 students or salary of 2000 employees. I need the Array. Array can store multiple values.
double salary []; double price[] ; int marks[];
If you see the Syntax it is in box style and as you know box can contain multiple things 😀
What is an Array ?
Array are oldest and simplest data structure. Used to store multiple items in one place and of same type e.g if u want to store multiple products in shopping , u can’t create n variables for this.
Good Parts of an Array?
- It provide Random Access Base Address + index * Size
- Cache Friendly - Cache is the memory near to processor. Usually processor pre-fetch the items near by the item. Cache Always bring the near by data (like contiguous data) Because elements are continuous so next index element always on the cache, so fast access. Array is having contiguous memory so array has advantage of cache friendliness, where linked list doesn’t have this advantage.
How to declare an One-D int Array in Java?
int x[]= {10,20,30}; int []x= {10,20,30}; int x[]= new int[]{10,20,30}; int x[]= new int[6];
Passing Function as an Argument to the Array , also return the Array From the Function
e.g. void updateArray(int [] array){ } e.g int [] getArray(){ int arr[] = new int[10]; return arr; }
Note: Array pass as an Reference to a Function , so if you change in the value it will be reflected to the calling point
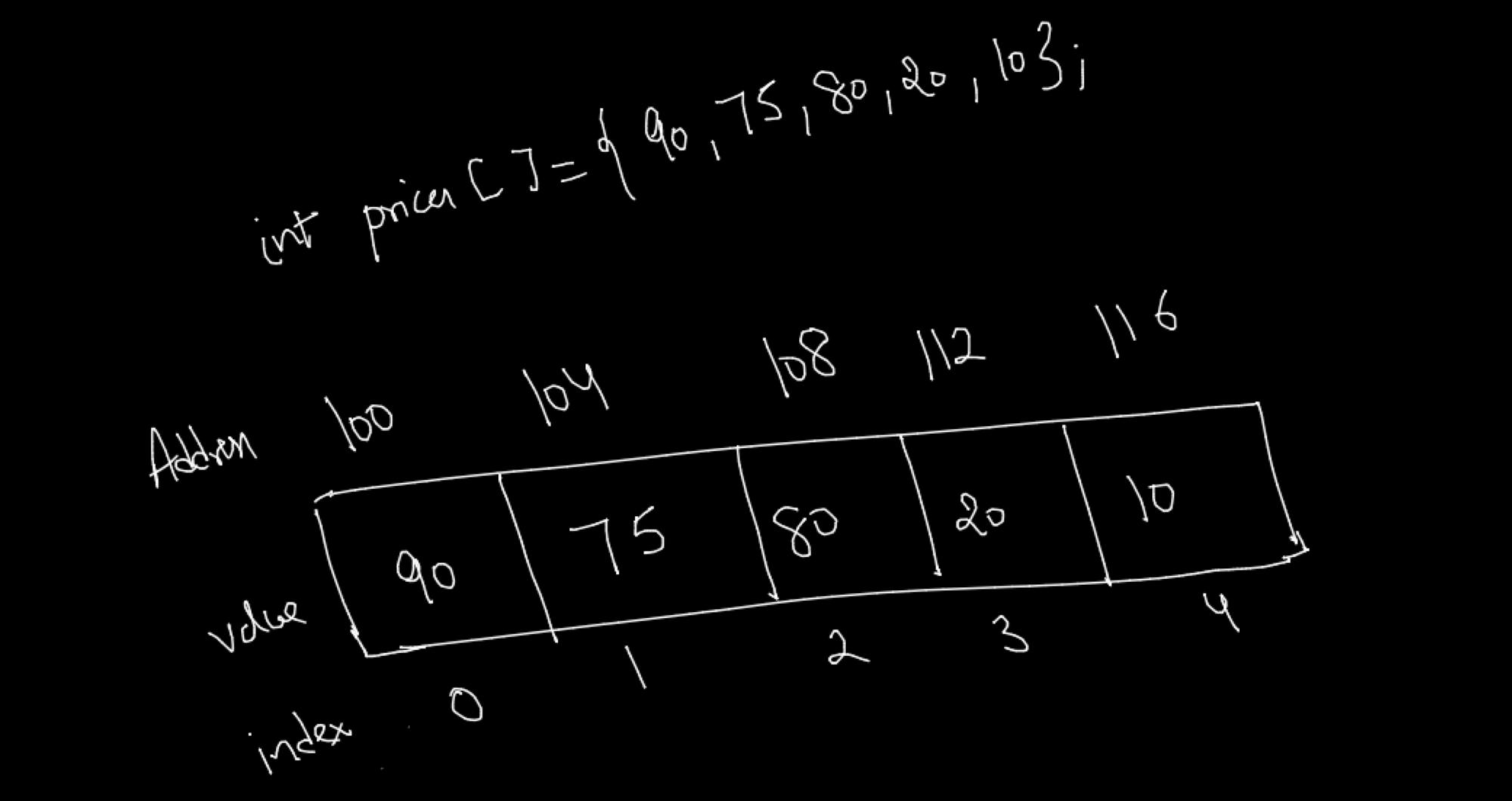
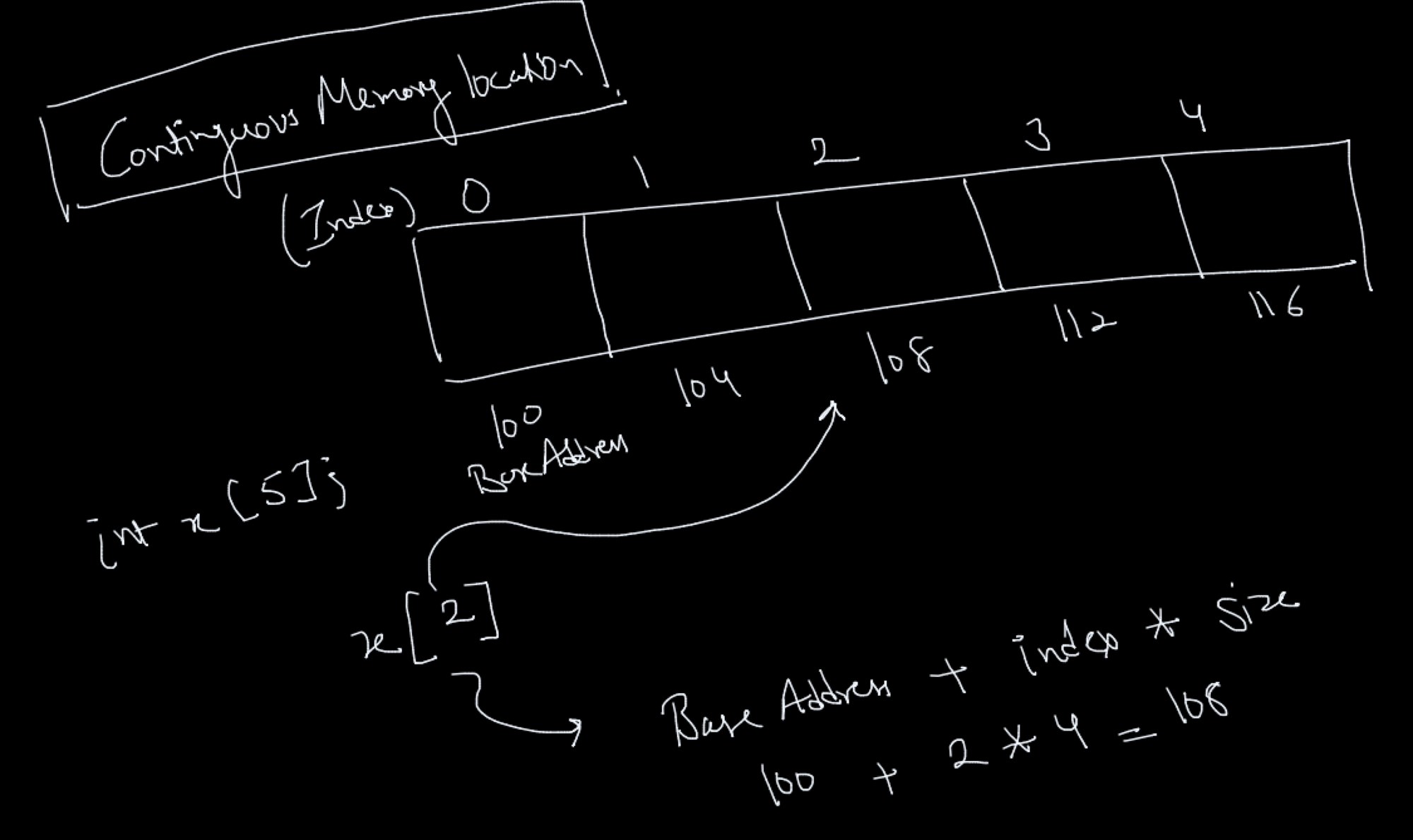
CRUD Operation in Array
CRUD Refers to Create, Read, Update and Delete Operations
Let's start with Create (A.K.A Insert Operation)
For Insertion the Logic is : Ask for the Position and the value where you want to insert the element in the Array. For Reference see the below image.
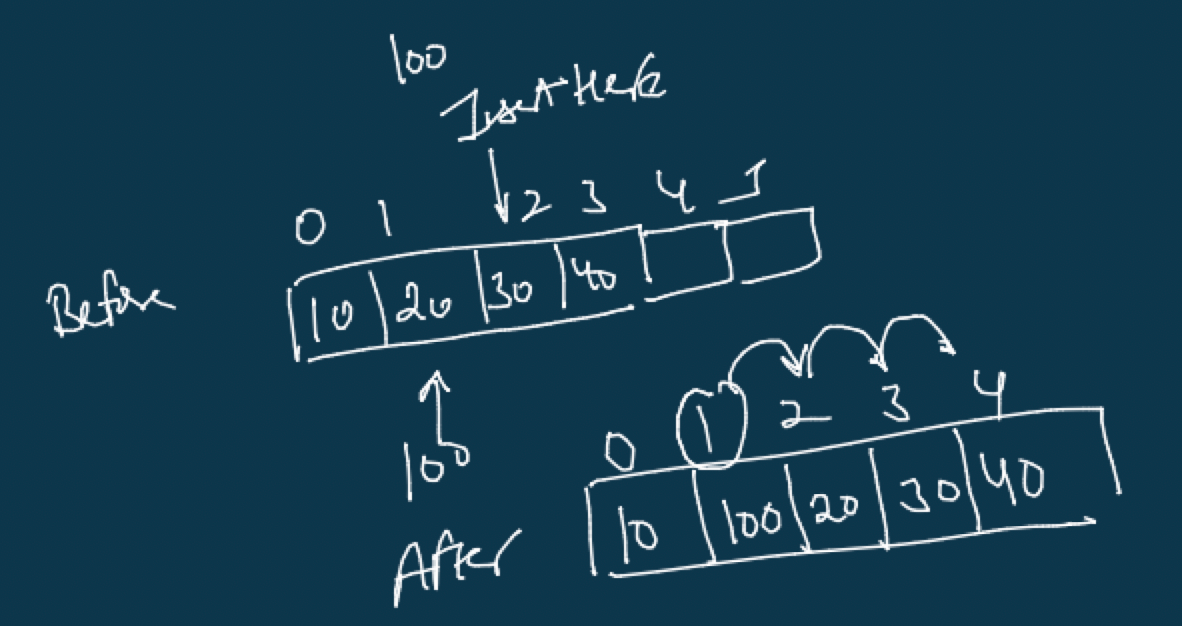
Let's start with the defination of the Function
int insert(int arr[] , int n , int element, int capacity, int position){ }
Function will recieve the Array (arr), number of elements in the array (n) , Total Capacity/Size of the Array (capacity) and the position where you want to insert the element (position).
In the insert function First start by Adding the Following Conditions
1. Check the number of element reach to the capacity of the array so u can't insert any new element in the array
if(n == capacity){ return n; // return the n (size of array) }
2. if the position is greater than the current size so can't allow to add the element in the array
if(position>n){ throw new RuntimeException("Position can't be greater than currentSize"); }
If the space is avaliable in the array so insert the element on the given position
Logic is first shift all the ith element to the i+1 position. e.g a[i+1] = a[i] it means ith element place to the i + 1 position
for(int i = n-1> ; i>=position; i—){ arr[i+1] = arr[i]; }
After the above loop you are on the position , and here u can place your element
arr[i] = element;
Finally the logic is
void insert(int index, int value){ //2.1 Adding Checks if(index>currentSize){ throw new RuntimeException("Position can't be greater than currentSize"); } if(currentSize == arr.length){ //throw new RuntimeException("Array Full"); System.out.println("Array Full Can't Add "); return; } // From Current Size till i reach to the index(position) move the element to the next index for(int i = currentSize-1; i>=index; i--){ arr[i+1] = arr[i]; } arr[index] = value ; // Element Inserted currentSize++; System.out.println("Element Added..."); print(); }
TC - O(N) . Best Case - if insert at last so it take Omega (1)
Now Start with Print Operation
First Define the Function print and travese array element one by one
void print(){ for(int element: arr){ System.out.println("Element "+element); } }
Now Move to the Remove Operation. First Start with the function defination
void remove(int index){}
Here define a function called remove and it take the index where you want to remove the element from the array
In the function first we check is array empty or not. If array is empty so raise an exception otherwise override the current index element with the next element till we each to the end of the array elements
for(int i = index; i<currentSize-1; i++){
arr[i] = arr[i+1]; //Opposite of Array Insert Operation
}
After this delete the last element by placeing Zero and reduce the array element size. Following is the Final Code , and the TC O(N)
void remove(int index){
if(currentSize==0){
//throw new RuntimeException("Array is Empty !");
System.out.println("Array is Empty Can't remove Further");
return ;
}
for(int i = index; i<currentSize-1; i++){
arr[i] = arr[i+1]; //Opposite of Array Insert Operation
}
arr[currentSize-1] = 0;
currentSize--;
System.out.println("Element Removed...");
print();
}
Let's move to the Next Operation - Search
Logic is to do the Linear Traverse from 0th index to last index and compare one by one element to the element you are looking for. if you found the match return the index of the element otherwise at the end of the loop return -1. Following is the code of Search and TC is O(N)
int search(int value){
for(int i = 0; i<arr.length; i++){
if(arr[i]== value){
System.out.println("Found "+value);
return i;
}
}
System.out.println("Not Found "+value);
return -1;
}
Let's move to the Next Operation - Update
Logic is to do the Search so basically calling the search function , if it return the index of the search element so on that index replace with the new value which you want to update
void update(int valueToSearch , int valueToUpdate){
int index = search(valueToSearch);
if(index ==-1){
return ;
}
else{
arr[index] = valueToUpdate;
System.out.println("Array Value Updated...");
print();
}
}
Following is the Final Code.
import java.util.Scanner;
/*
In this Program You will learn CRUD Operation in Array
*/
class ArrayBasics01{
int arr[] ;
int currentSize; // Used to indentify how many elements currently in the array
// 1. Create Constructor for Initalize
ArrayBasics01(int capacity){
this.arr = new int[capacity];
int currentSize = 0;
}
// 2. Create Insert Function
void insert(int index, int value){
//2.1 Adding Checks
if(index>currentSize){
throw new RuntimeException("Position can't be greater than currentSize");
}
if(currentSize == arr.length){
//throw new RuntimeException("Array Full");
System.out.println("Array Full Can't Add ");
return;
}
// From Current Size till i reach to the index(position) move the element to the next index
for(int i = currentSize-1; i>=index; i--){
arr[i+1] = arr[i];
}
arr[index] = value ; // Element Inserted
currentSize++;
System.out.println("Element Added...");
print();
}
// 3. Print
void print(){
for(int element: arr){
System.out.println("Element "+element);
}
}
// 4. remove
void remove(int index){
if(currentSize==0){
//throw new RuntimeException("Array is Empty !");
System.out.println("Array is Empty Can't remove Further");
return ;
}
for(int i = index; i<currentSize-1; i++){
arr[i] = arr[i+1]; //Opposite of Array Insert Operation
}
arr[currentSize-1] = 0;
currentSize--;
System.out.println("Element Removed...");
print();
}
// 5. Search in an Array (Linear Search)
int search(int value){
for(int i = 0; i<arr.length; i++){
if(arr[i]== value){
System.out.println("Found "+value);
return i;
}
}
System.out.println("Not Found "+value);
return -1;
}
// 6. Update Operation in Array
void update(int valueToSearch , int valueToUpdate){
int index = search(valueToSearch);
if(index ==-1){
return ;
}
else{
arr[index] = valueToUpdate;
System.out.println("Array Value Updated...");
print();
}
}
public static void main(String[] args) {
ArrayBasics01 opr = new ArrayBasics01(5);
Scanner scanner = new Scanner(System.in);
int choice;
int index;
int value;
while(true){
System.out.println("Array Operations");
System.out.println("1. Array Insert at Specific Position");
System.out.println("2. Array Delete From Specific Position");
System.out.println("3. Search in Array");
System.out.println("4. Print the Array");
System.out.println("5. Update the Array");
System.out.println("6. Exit");
System.out.println("Enter the Choice");
choice = scanner.nextInt();
ArrayOperations enumChoice = ArrayOperations.values()[choice-1];
switch(enumChoice){
case INSERT:
System.out.println("Enter the Index");
index = scanner.nextInt();
System.out.println("Enter the Value");
value = scanner.nextInt();
opr.insert(index, value);
break;
case DELETE:
System.out.println("Enter the Index Where you want to Remove the Value");
index = scanner.nextInt();
opr.remove(index);
break;
case SEARCH:
System.out.println("Enter the Value to Search");
value = scanner.nextInt();
opr.search(value);
break;
case UPDATE:
System.out.println("Enter the Value to Search");
value = scanner.nextInt();
System.out.println("Enter the Value to Update");
int value2 = scanner.nextInt();
opr.update(value, value2);
break;
case PRINT:
opr.print();
break;
case EXIT:
return ;
default:
System.out.println("Wrong Choice...");
}
}
}
}
// Enum Represent the Group of Constants , Enum Introduce in Java 1.5
enum ArrayOperations{
INSERT, DELETE, SEARCH, PRINT, UPDATE, EXIT;
}
That's All Folks  , Will Catch you in the next Tutorial 😀